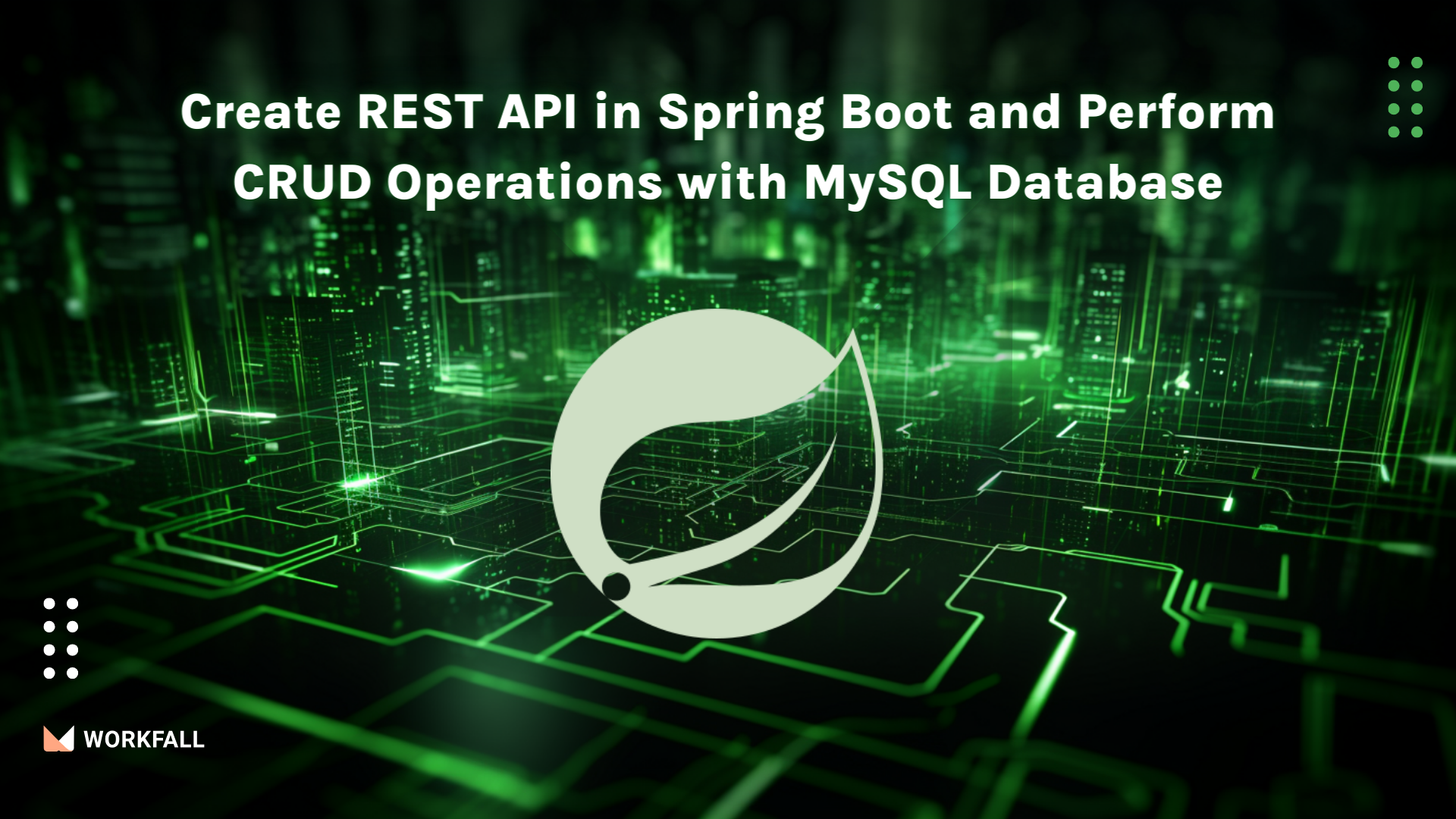
In this blog, we will cover:
- What are CRUD Operations?
- What is Spring Boot?
- What is MySQL Database?
- What is REST API
- Hands-On
- Conclusion
What are CRUD Operations?
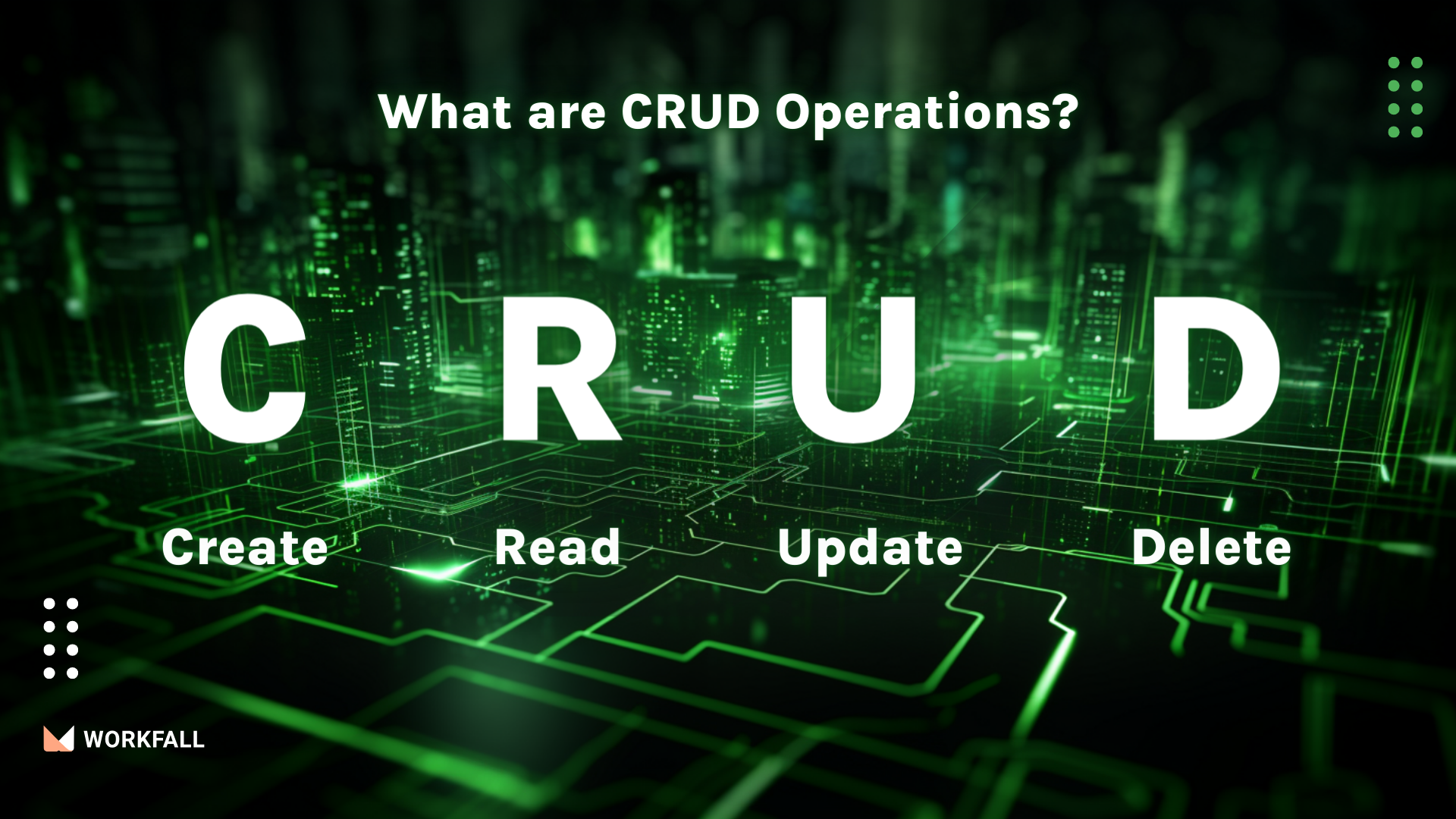
CRUD represents Create, Read/Retrieve, Update, and Delete – fundamental actions on persistent storage, aligned with HTTP methods used in web development and database management:
– POST: Establishes a fresh resource.
– GET: Retrieves/reads a resource.
– PUT: Modifies an existing resource.
– DELETE: Removes a resource.
As the name suggests:
CREATE Operation: Enacts the INSERT statement to create a new record.
READ Operation: Retrieves table entries determined by the provided input parameter.
UPDATE Operation: Executes an update statement on the table, based on the input parameter.
DELETE Operation: Removes a designated row from the table, also depending upon the input parameter.
What is Spring Boot?
Spring Boot is a framework built on top of Spring, including all its features. It’s popular among developers because it offers a quick production-ready setup, allowing them to concentrate on coding instead of dealing with configurations. With its microservice approach, creating production-ready applications is fast and easy.
What is MySQL Database?
MySQL is a widely used open-source relational database management system. It efficiently stores and retrieves data for software applications, websites, and more. Known for its reliability and speed, MySQL supports various data types, transactions, and complex queries. It offers both client-server and embedded modes, allowing it to be integrated into different setups. With a large user community and extensive documentation, MySQL is a popular choice for businesses and developers seeking a robust and scalable database solution.
What is REST API?
A REST API (Representational State Transfer Application Programming Interface) is a set of rules that enable different software applications to communicate and interact over the Internet. It follows the principles of REST, using standard HTTP methods like GET, POST, PUT, and DELETE to perform operations on resources such as data or services. REST APIs use URLs to identify resources and return data in formats like JSON or XML. This architecture promotes scalability, modularity, and simplicity, allowing diverse systems to work together seamlessly. REST APIs are widely used for building web services, mobile apps, and other distributed software applications.
Hands-On
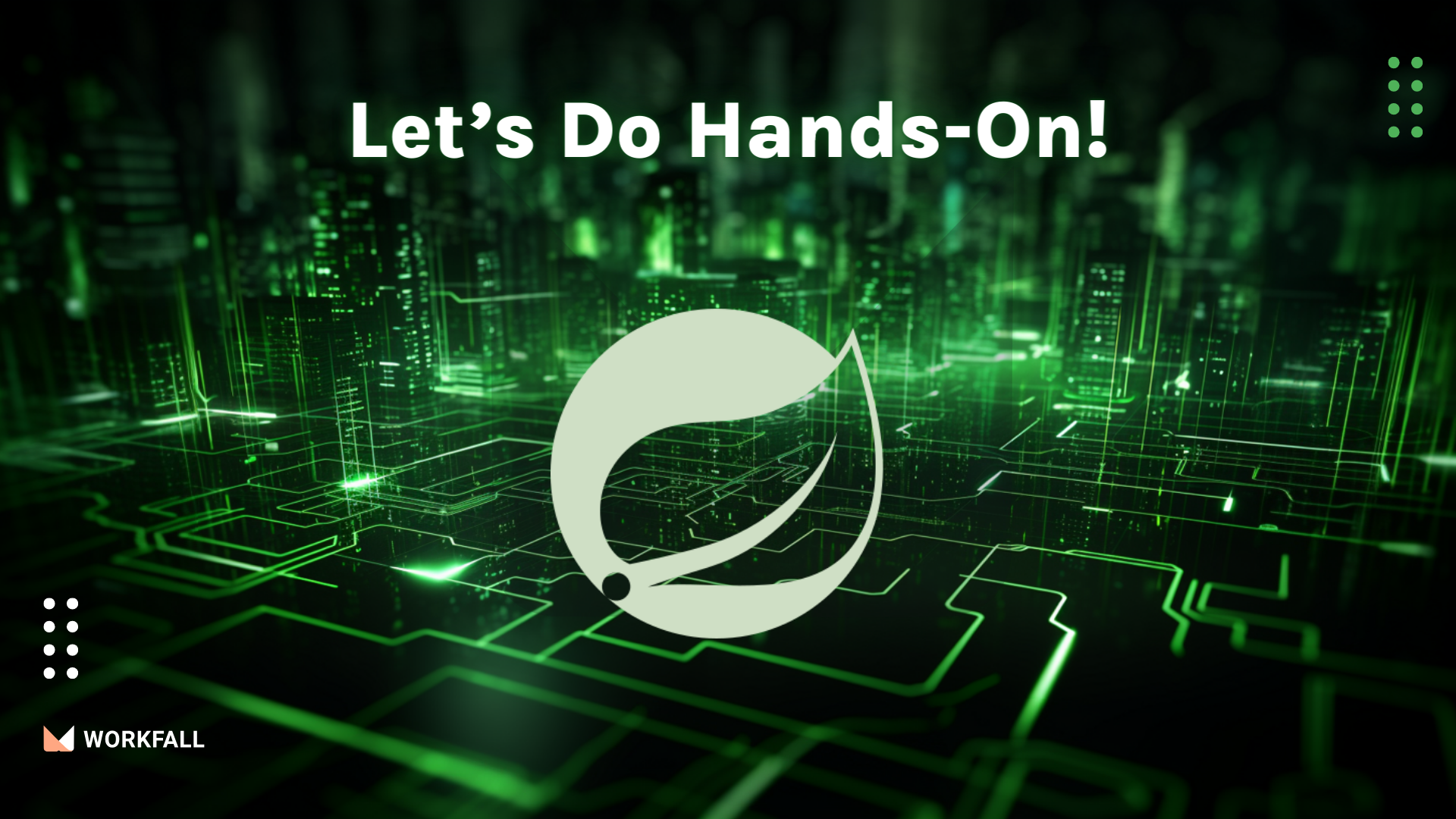
Required Installations:
To perform the demo, you require the following installations:
- Java: Java is a widely used, object-oriented programming language known for its platform independence and versatility.
- IntelliJ: IntelliJ IDEA is an integrated development environment (IDE) for software development, primarily used for Java projects.
- Postman: Postman is a popular API development and testing tool that simplifies the process of working with APIs.
In this hands-on, we will discover how to build a REST API with Spring Boot. This API allows us to do basic things like creating, reading, updating, and deleting data in a MySQL database. We will make use of handy tools such as Spring Boot’s CrudRepository and Lombok.
To start, we’ll initialize the new Spring Boot code using Spring Boot Initializr and then open it up in IntelliJ IDE. We will neatly divide our tasks into different sections and make sure our code follows good practices for writing clean and organized programs.
As we move forward, we will include all the necessary libraries in our project and start writing our code. Once we’ve coded everything, we need to test it to make sure it works correctly. We will make use of the Postman tool to accomplish this. We’ll also take a look at the database itself using SELECT queries to be sure that the data we put in is actually there.
This tutorial makes the process of setting up a project in IntelliJ IDE and creating a Spring Boot REST API much simpler and easier to understand.
Open Spring Initializr in your browser.
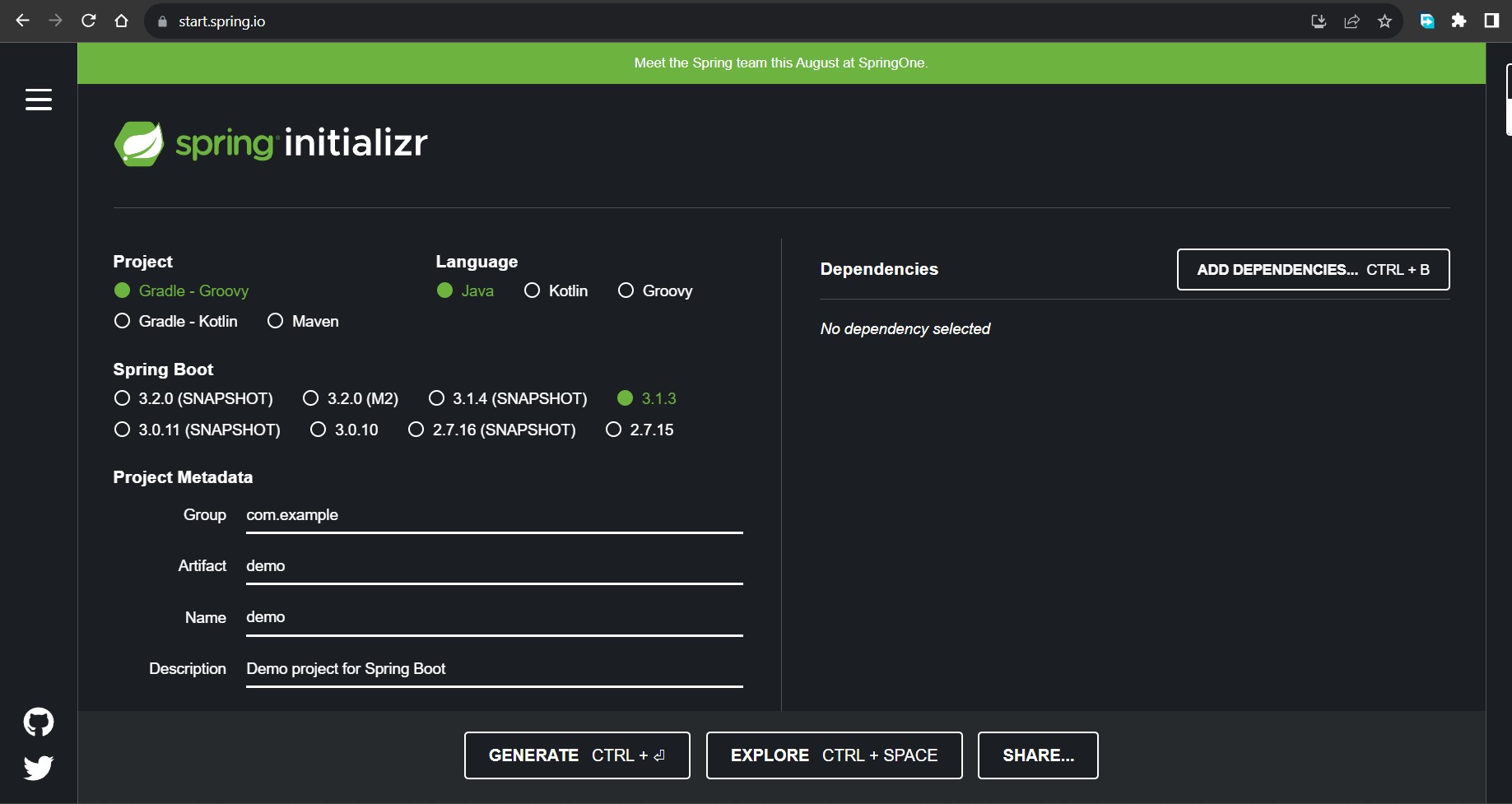
Click on the “Add Dependencies
” button.
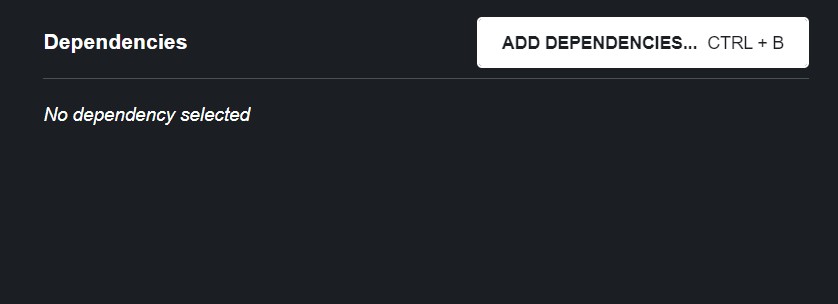
Search for the “Spring Web
” library.
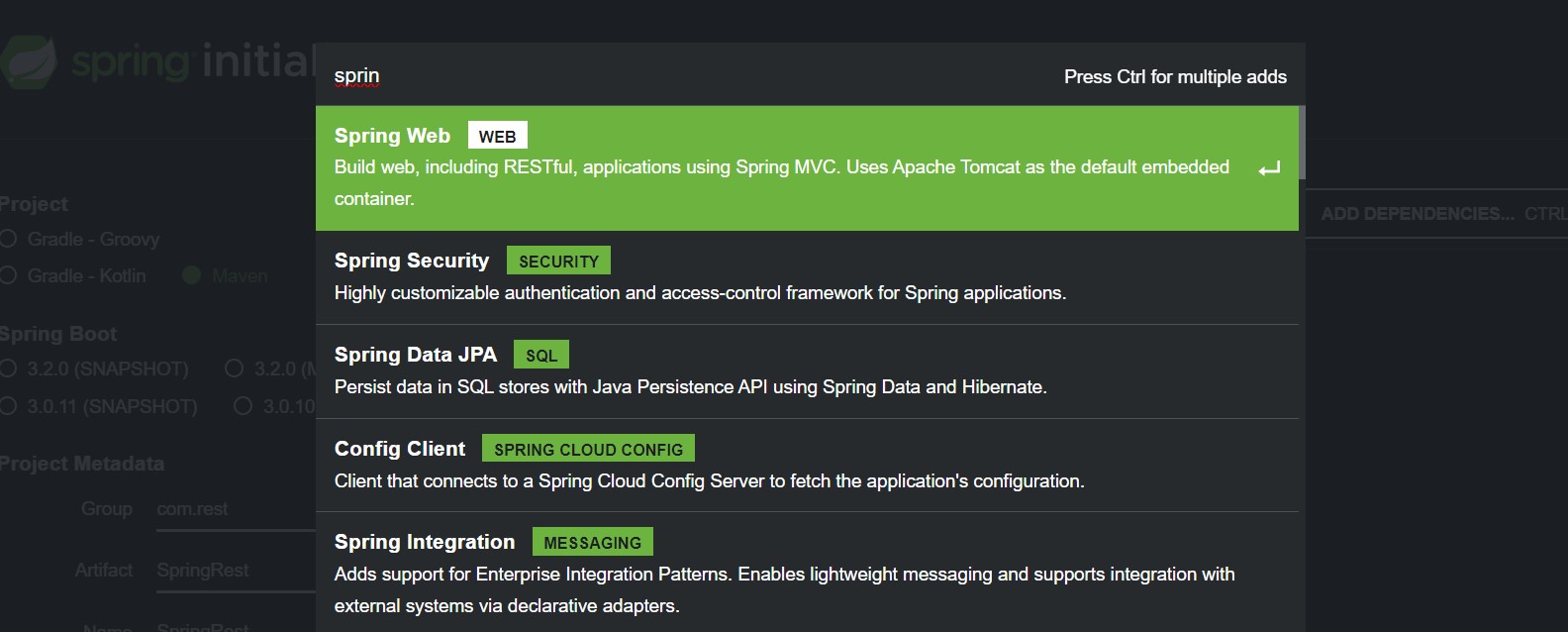
Click on the library name and it will get added to your project.
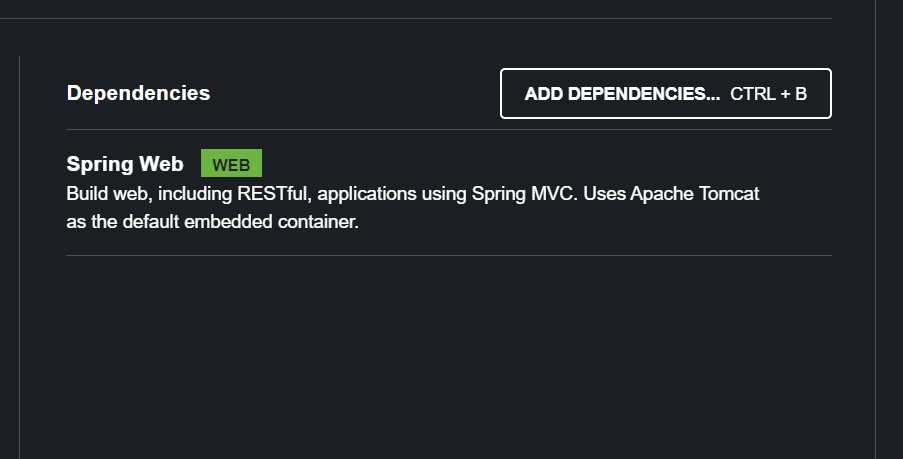
Fill in all the details accordingly and last click on the “Generate
” button below. This will download your Spring Boot project in zip format.
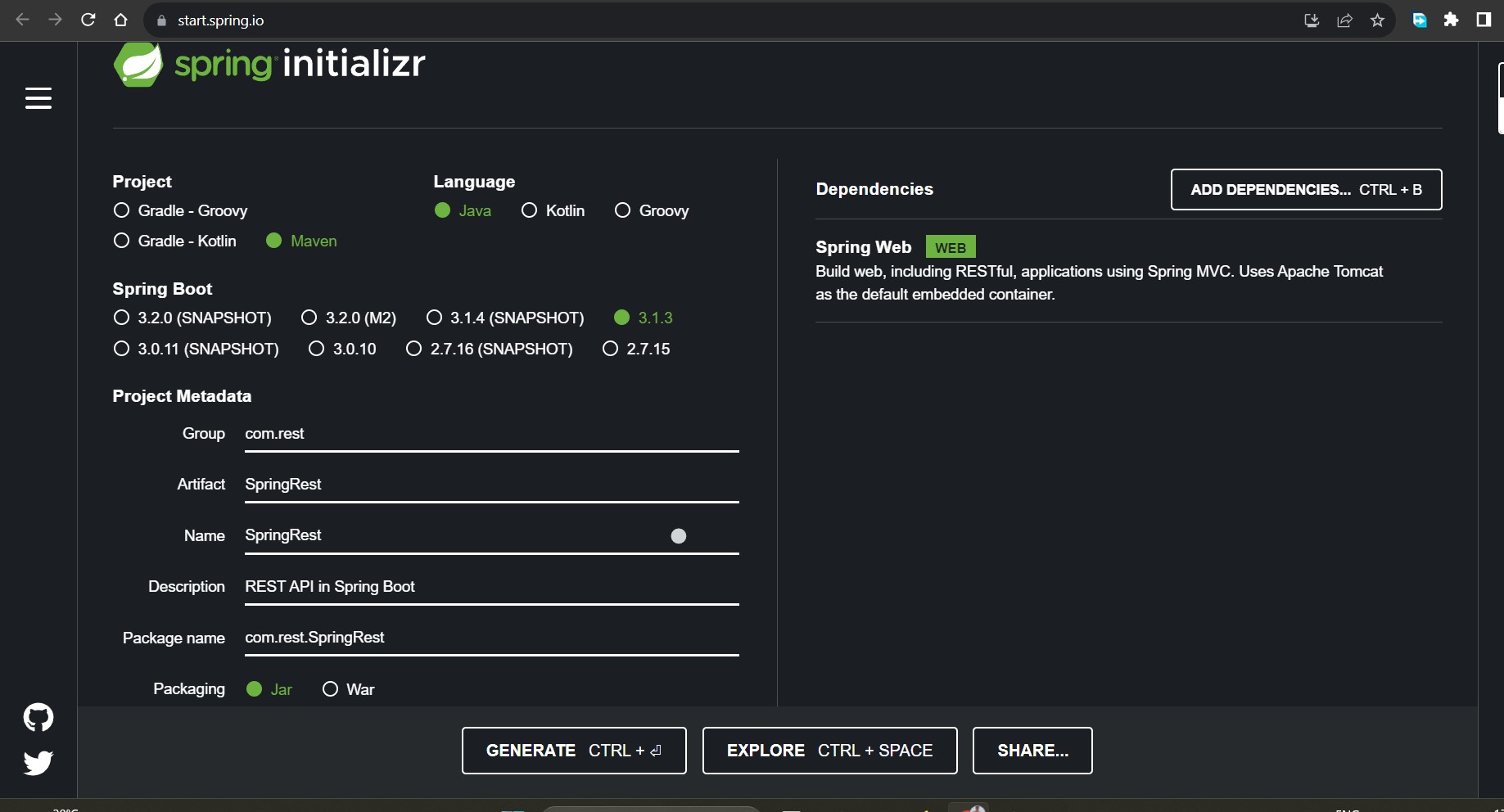
Extract the downloaded zip file then open the IntelliJ IDE and click on file>open.
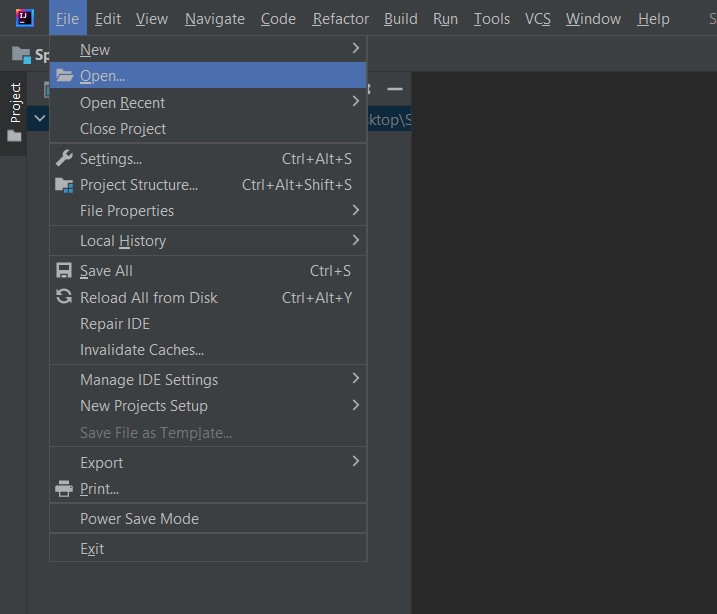
Open the extracted folder in the IDE by selecting the path of the folder.
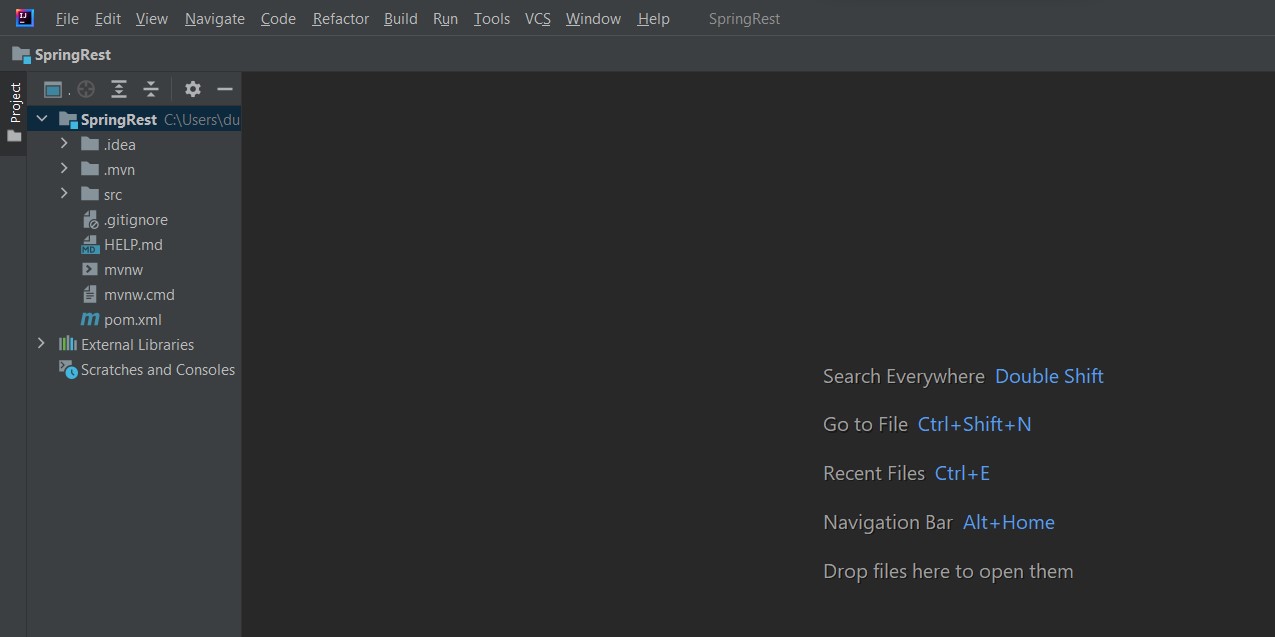
By now, the Spring Boot project has been set up as shown in the image. On the left, you have the project’s file structure, and in the middle, there’s the code editor.
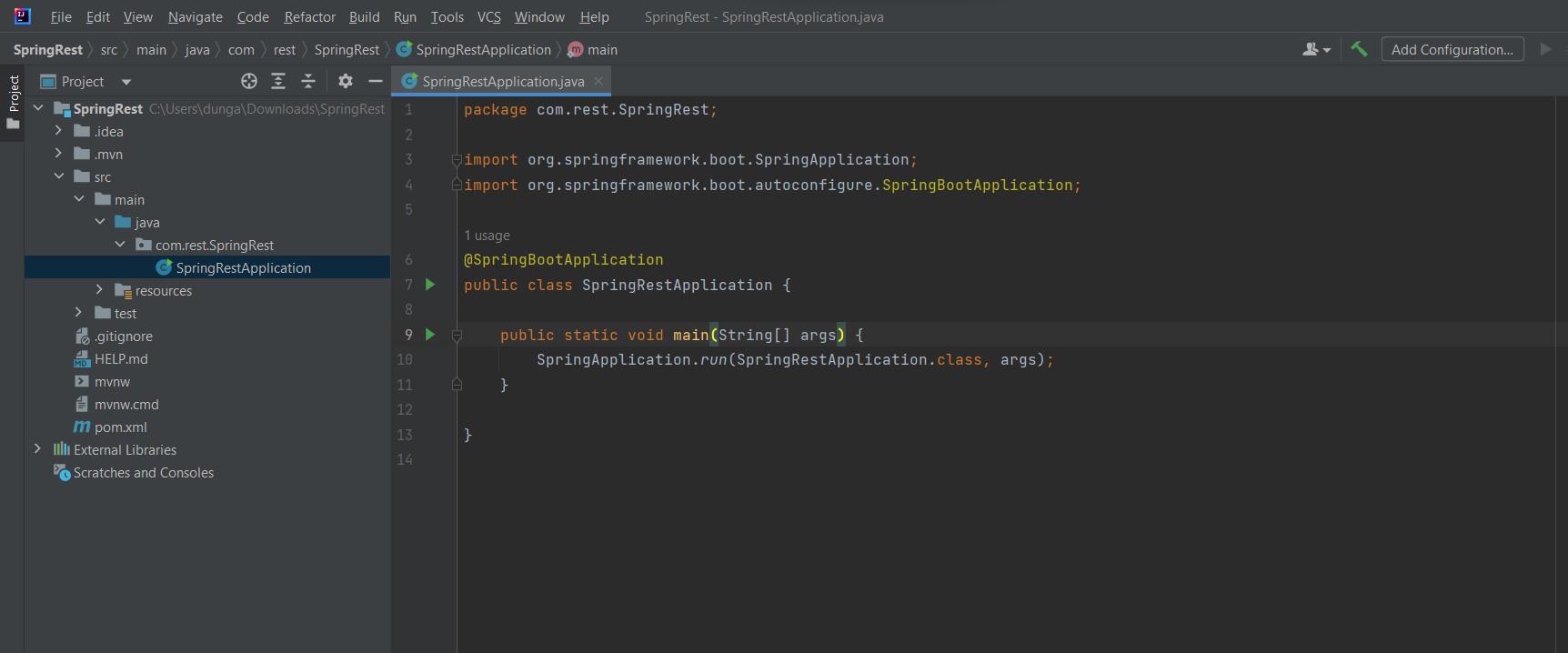
Add the following dependency to the “pom.xml
” file
- Spring Web
- MySQL Database
- Lombok
- Spring Data JPA
The final “pom.xml
” file should look like as shown in the image below.
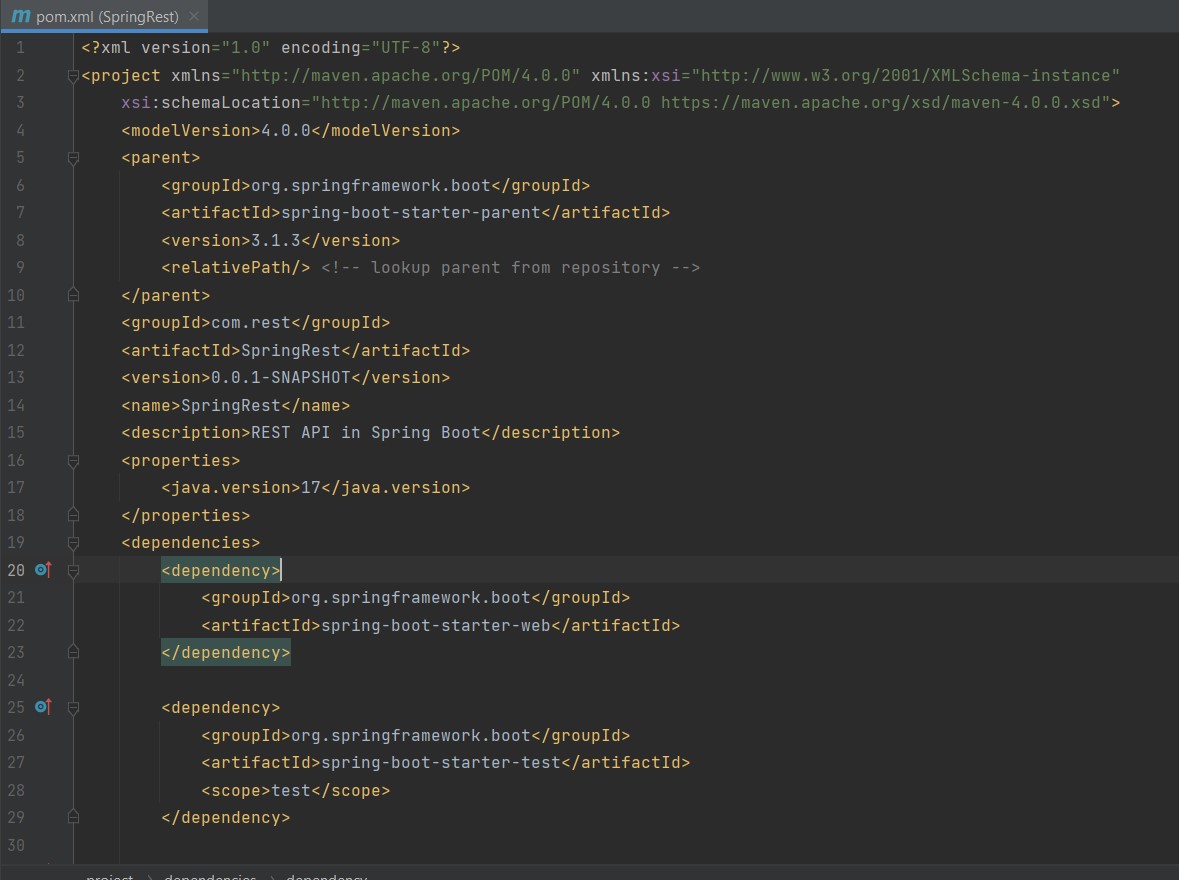
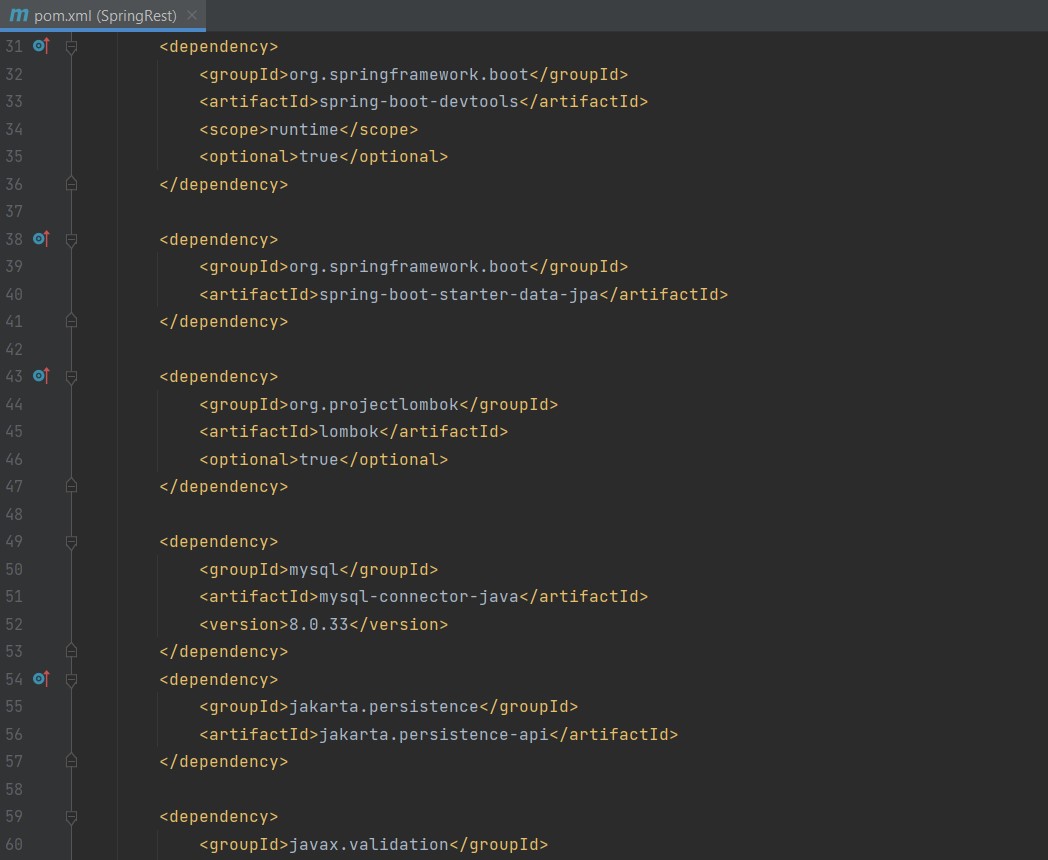
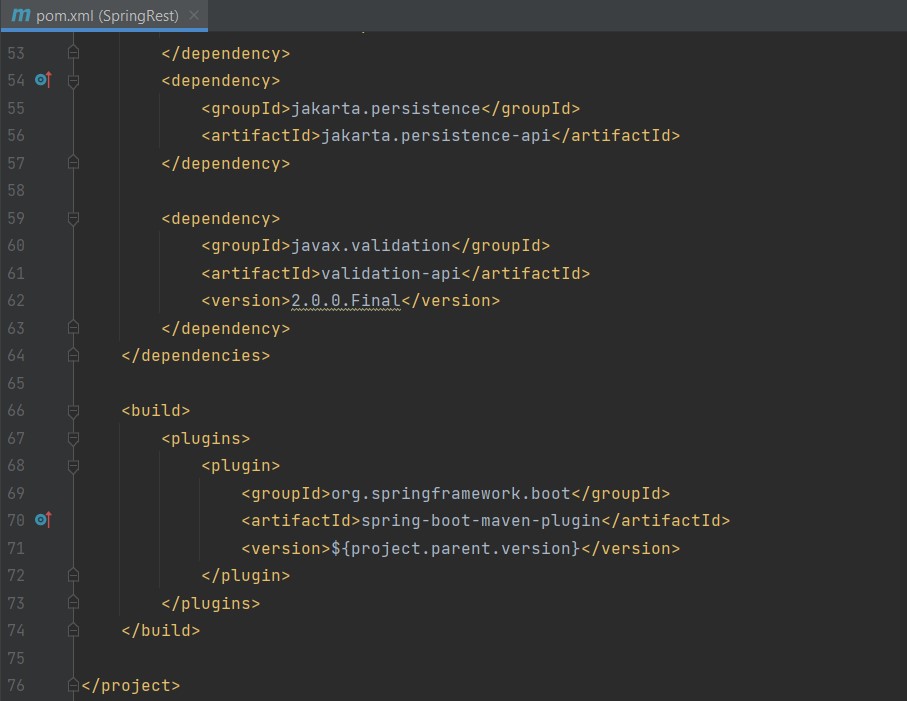
Right-click on “SpringRest
” package and create a new Package inside it.
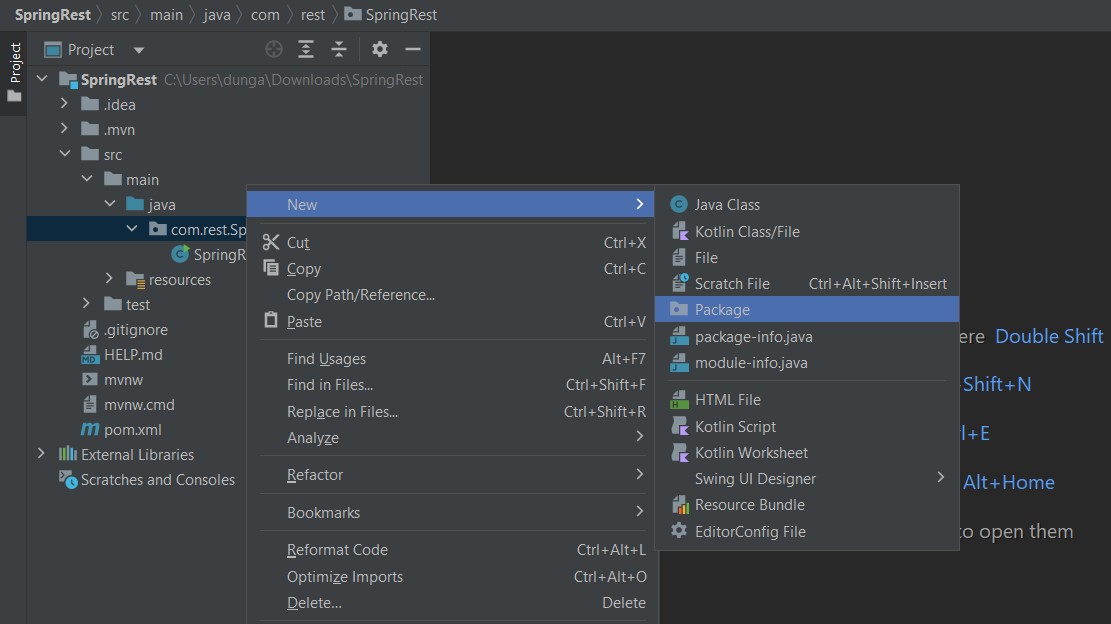
Name the package “entity
” and create a POJO class inside this newly created package by right-clicking the package as shown in the image below.
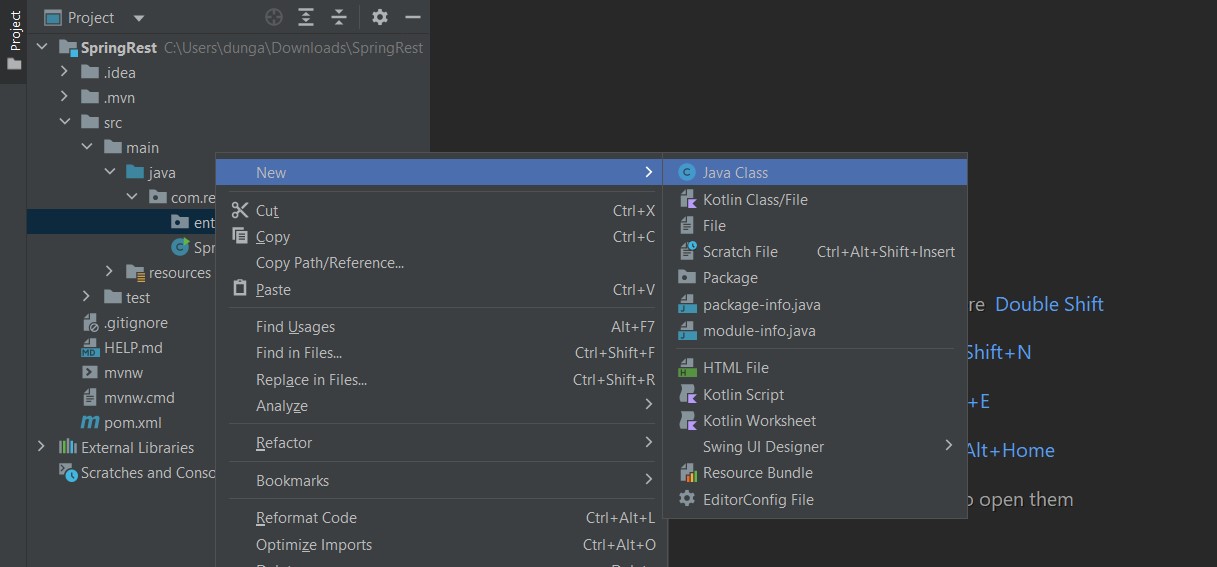
Name the file as a Student and save the file.
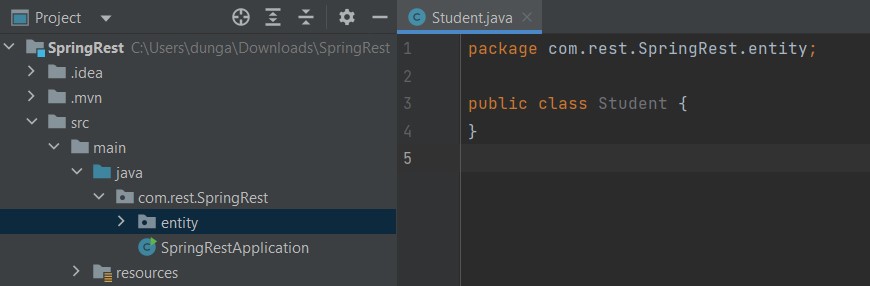
Write the below-provided code inside the Student.java
file.
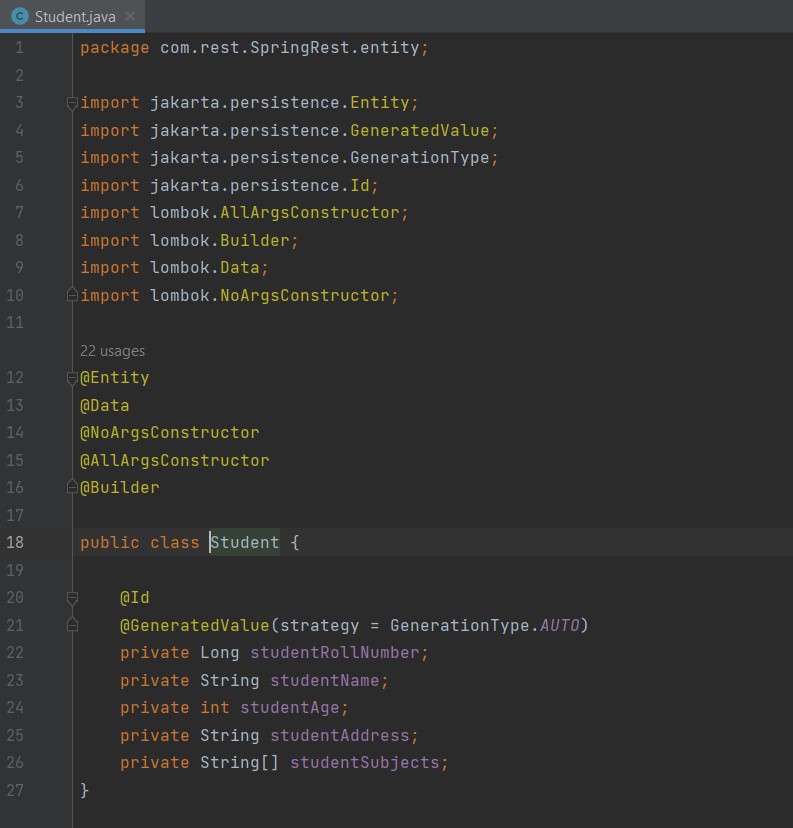
Create another package inside the “SpringRest
” package and name it as “repository
”.
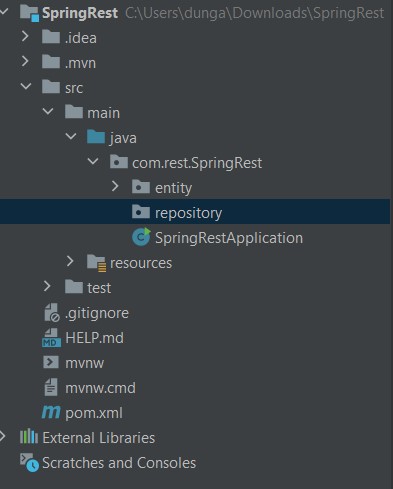
In the “repository
” package, create an interface named “StudentRepository
” that extends the “CrudRepository
” interface. Below is the code for the “StudentRepository.java
” file:
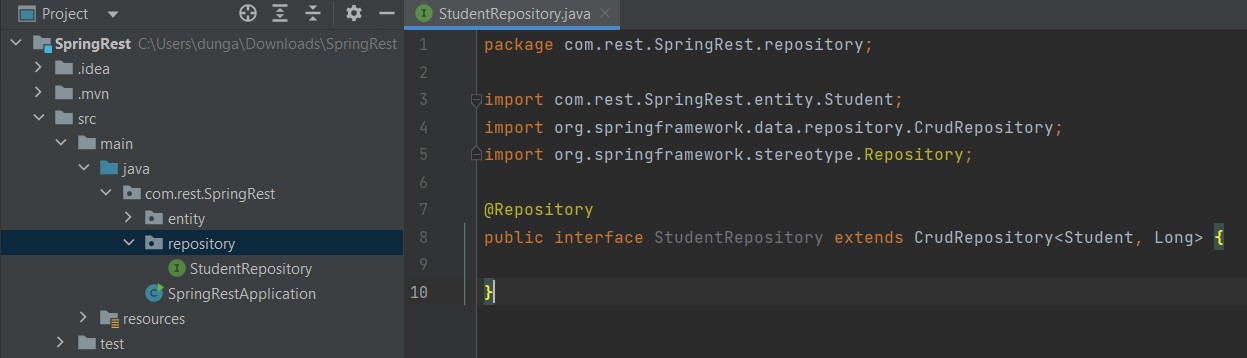
Create another package inside the “SpringRest
” package and name it “service
”.
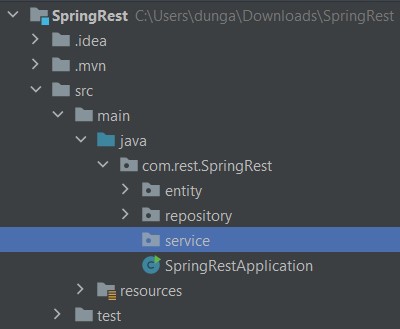
In the “service
” package, create an interface named “StudentService.java
“
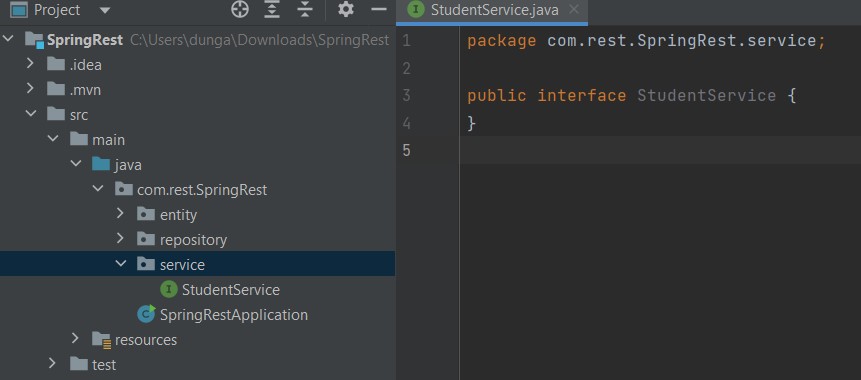
Write the below-provided code in the newly created interface “StudentService.java
”:
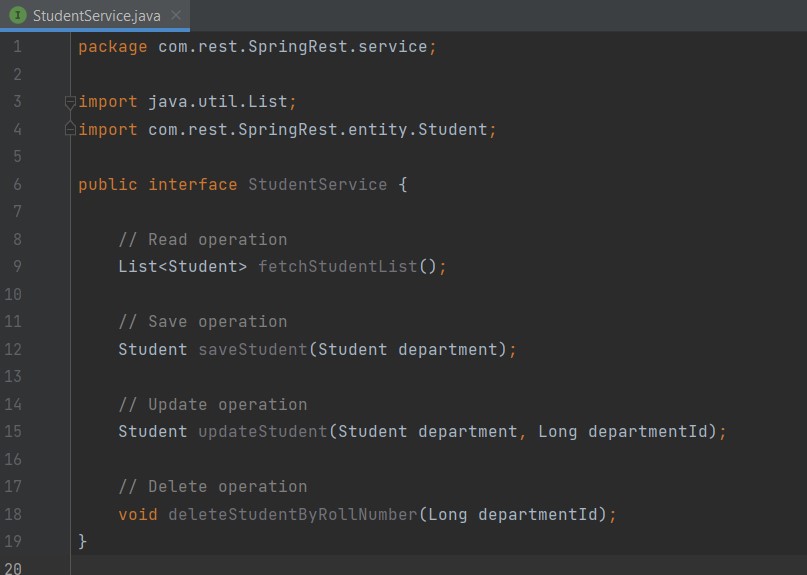
In the “service
” package, create a java class named “StudentServiceImpl.java
“
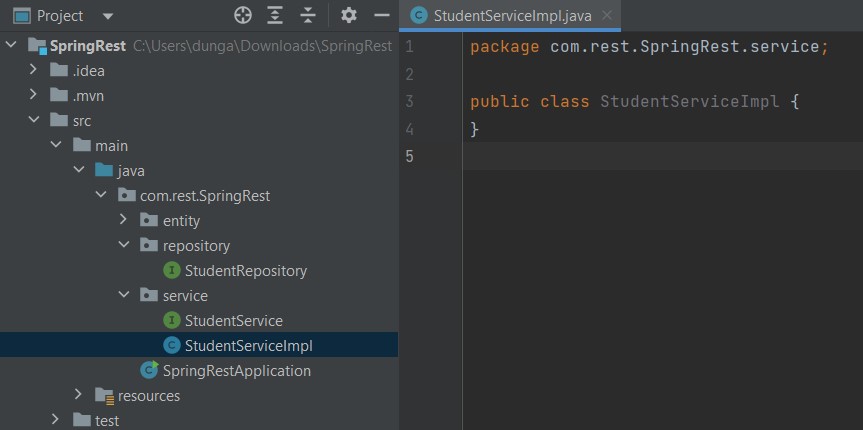
Write the below-provided code inside the “StudentServiceImpl.java
” file:
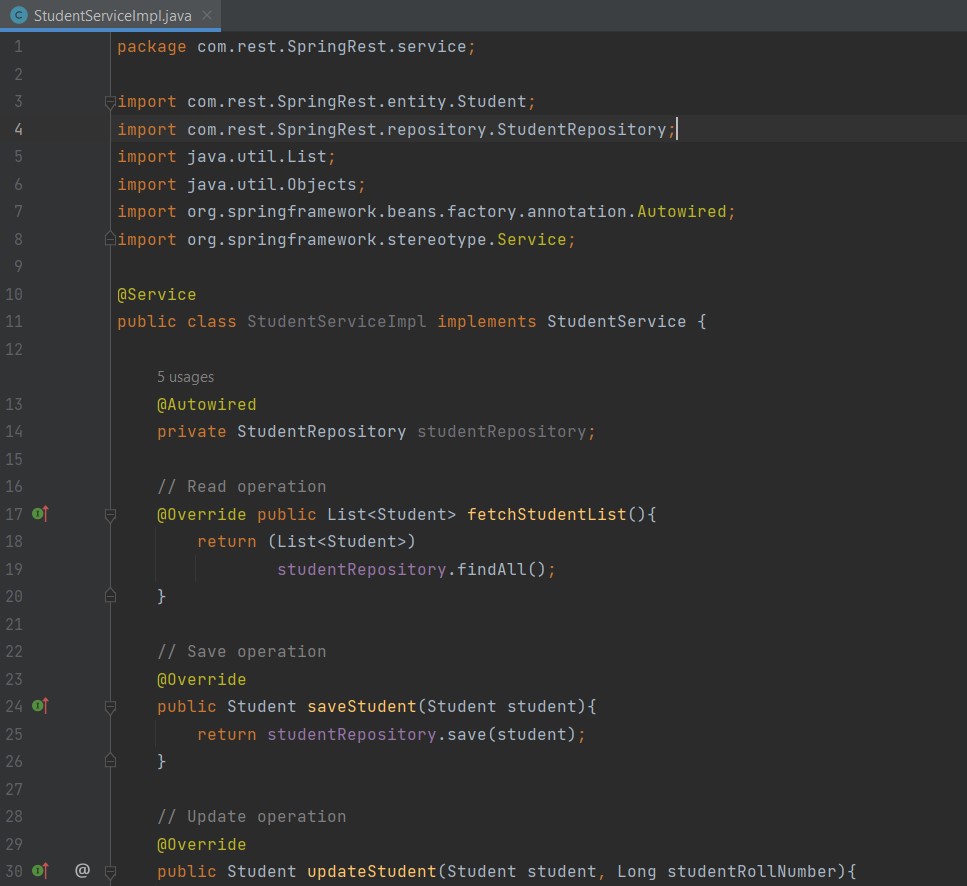
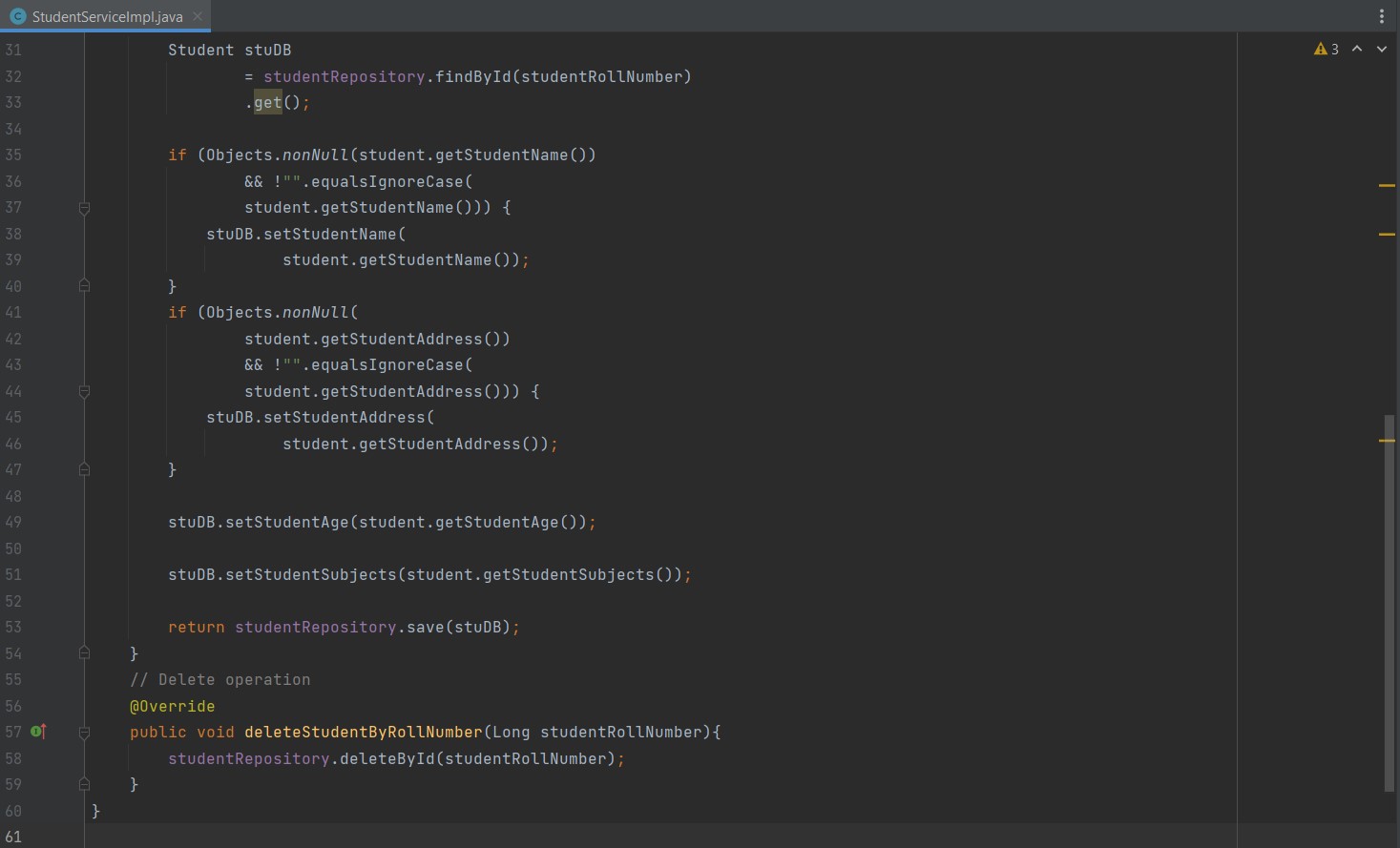
Create another package inside the “SpringRest
” package and name it as “controller
”.
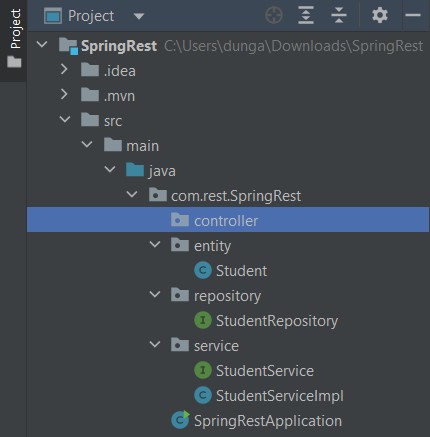
Inside the “controller
” package creates one class named StudentController.java
.
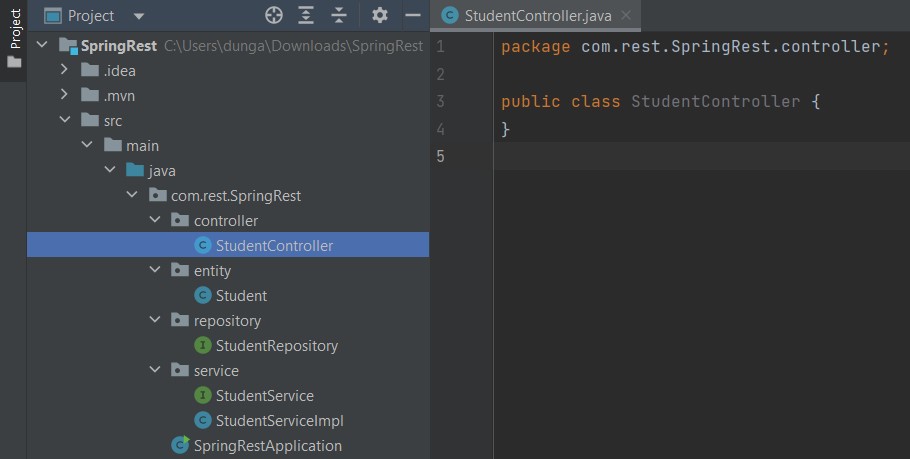
Write the below-provided code inside the “StudentController.java
” file:
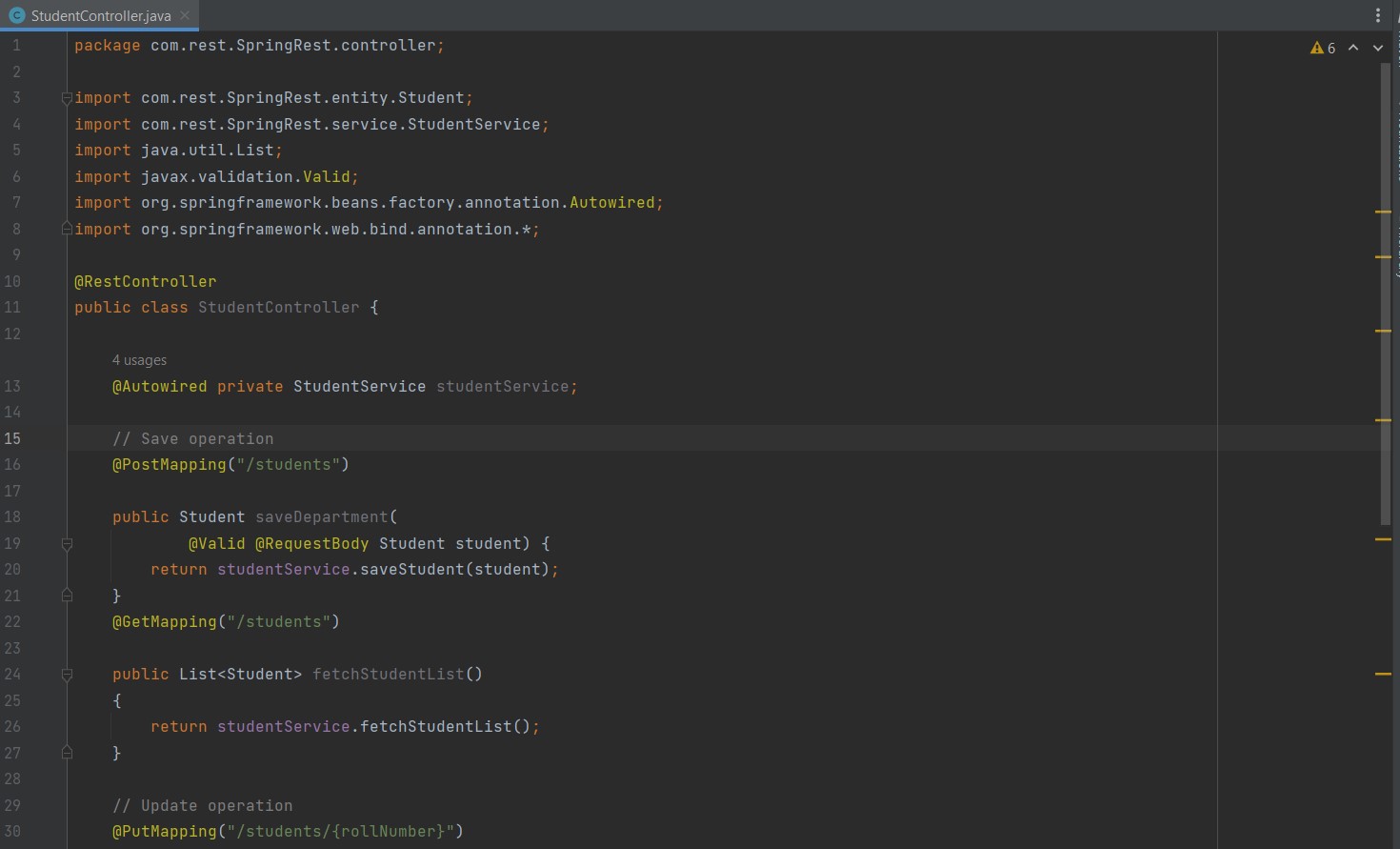
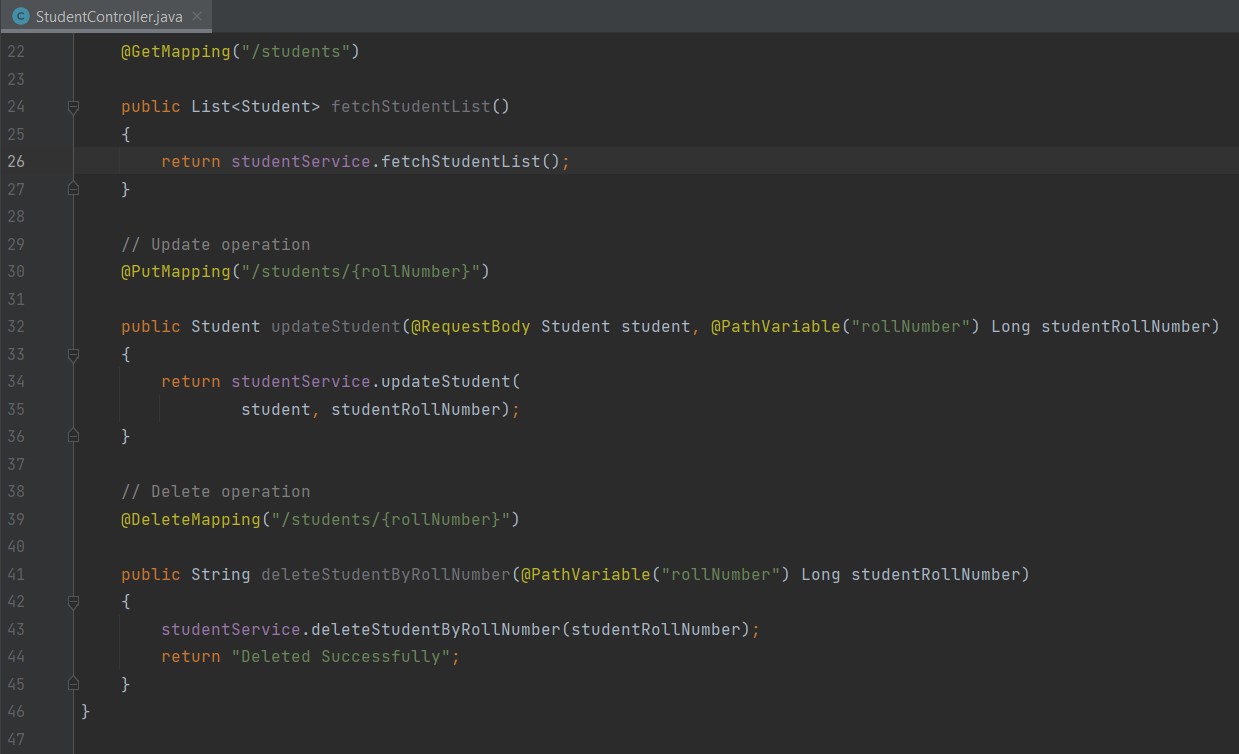
Now, write the below-provided code inside the application.properties file inside the resources folder. Make sure to write your own MySQL username and password in the respective fields.
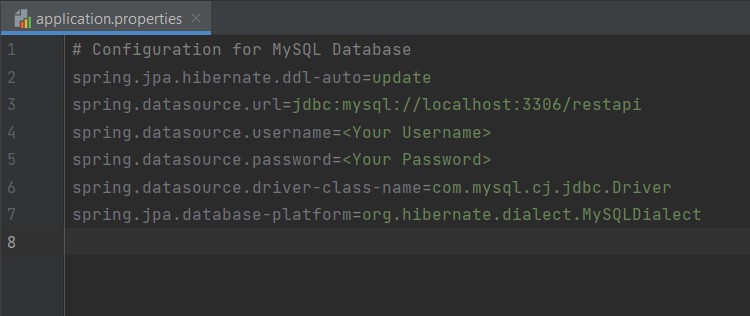
Before running the application, let’s first create a database in MySQL with the name “restapi
” using the query shown in the image below.
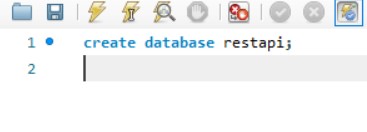
To run the application, right-click on the “SpringRestApplication
” file and click on the option as shown in the image below.
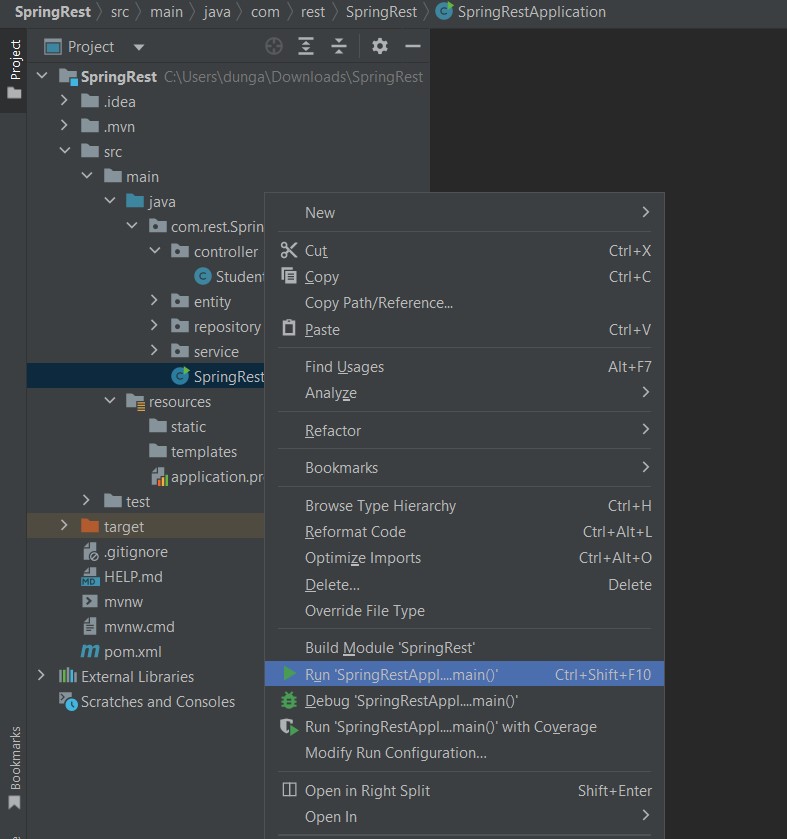
Now, let’s go ahead and test our application using the Postman tool.
Endpoint 1: POST – http://localhost:8080/students/
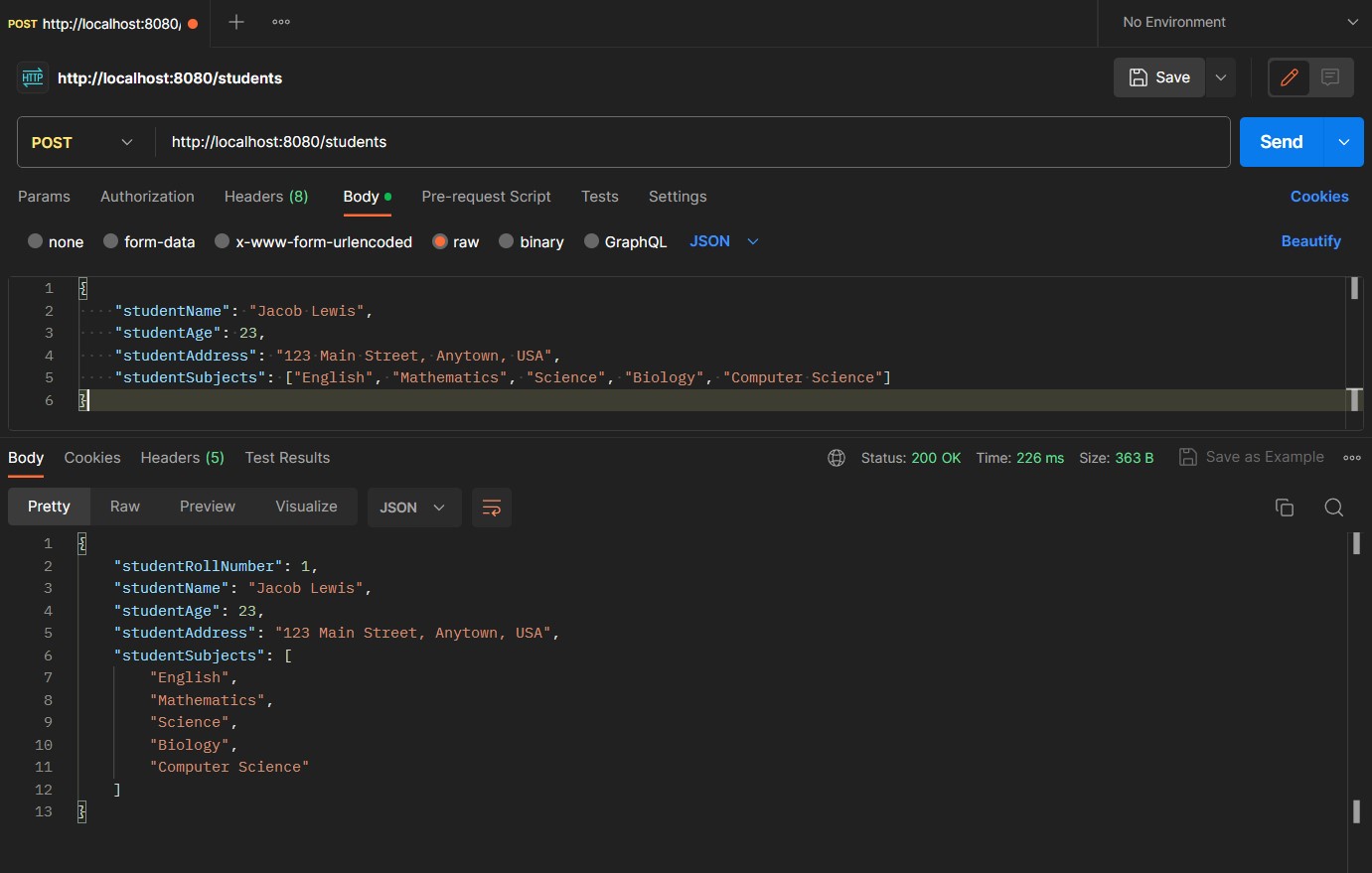
Check the student table in the database by running the query as shown in the image below.
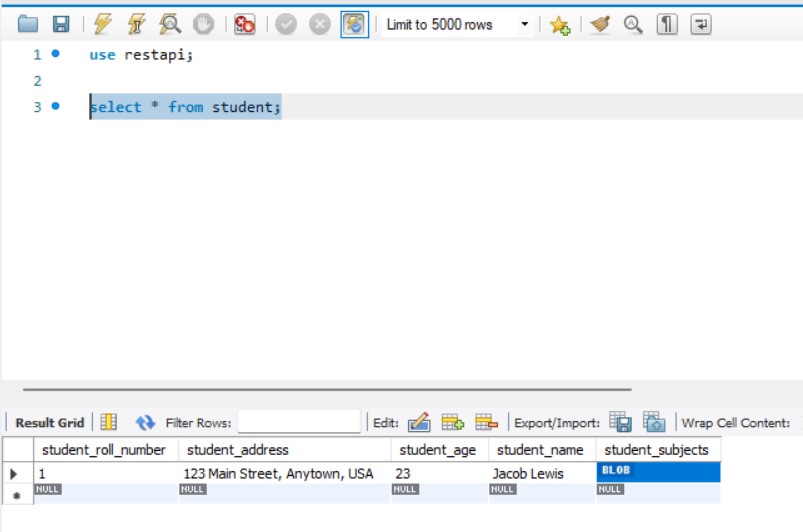
Endpoint 2: GET – http://localhost: 8080/students/
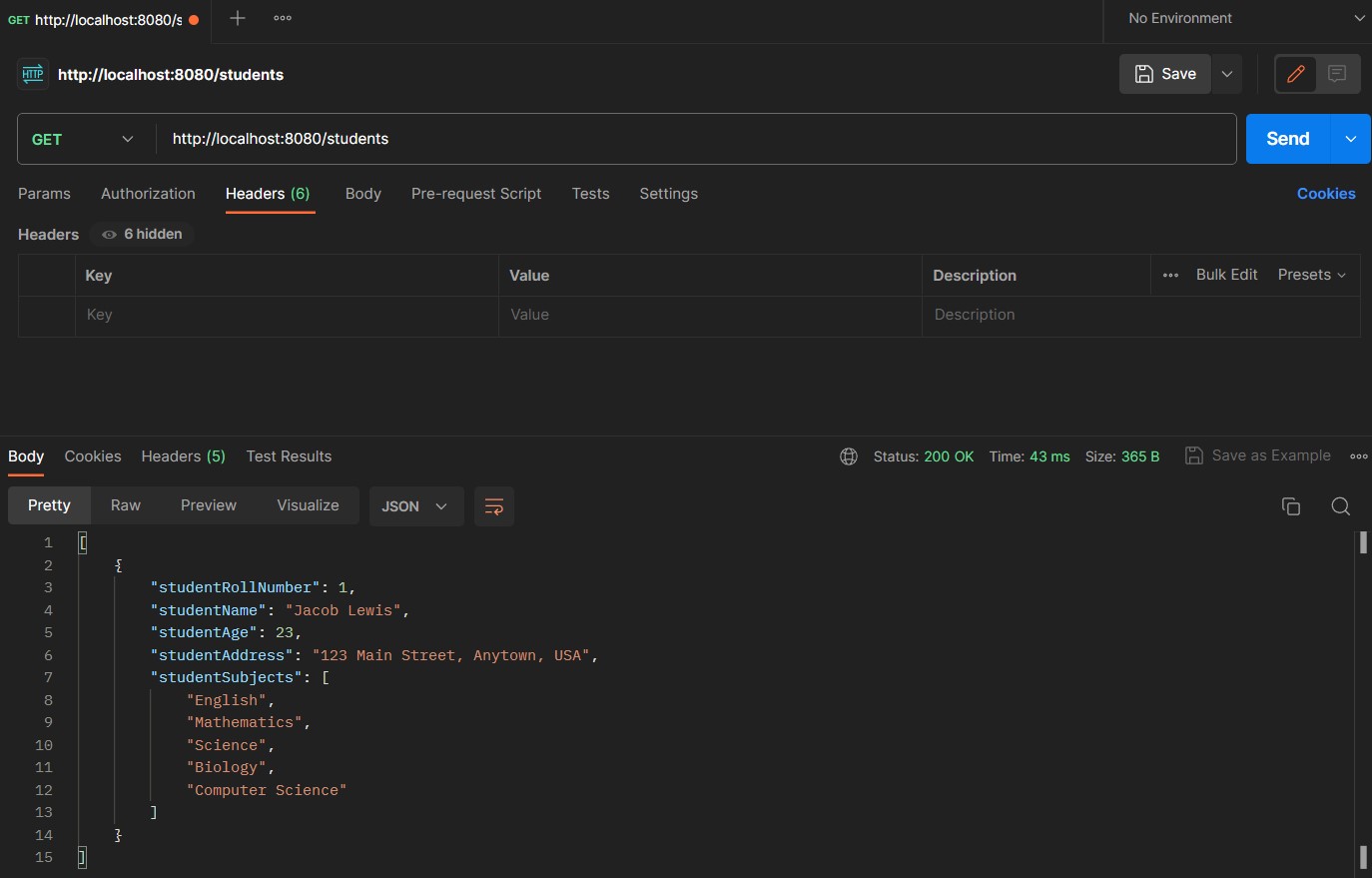
Endpoint 3: PUT – http://localhost: 8080/students/1
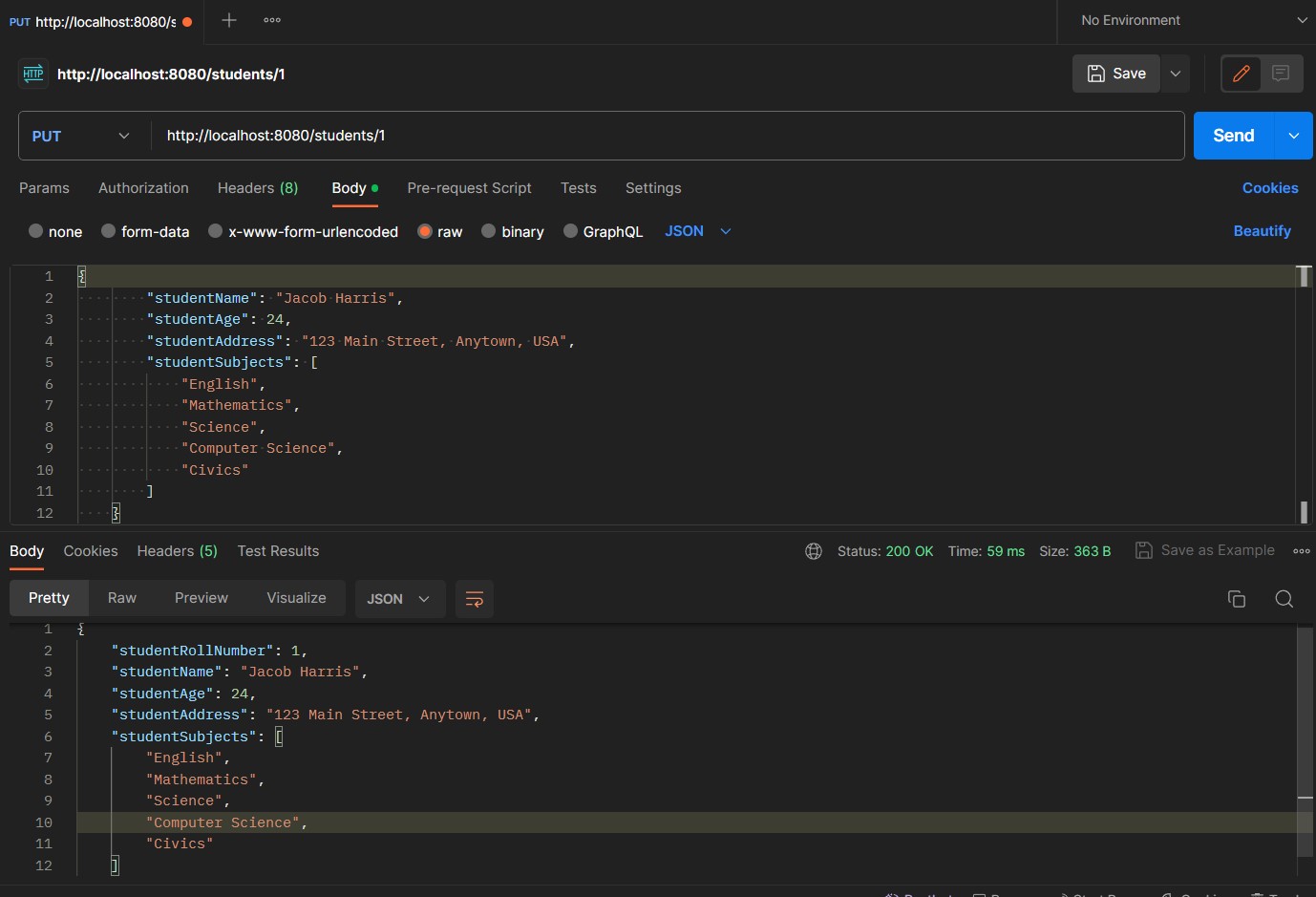
Check the database for the updated value using the below-shown query.
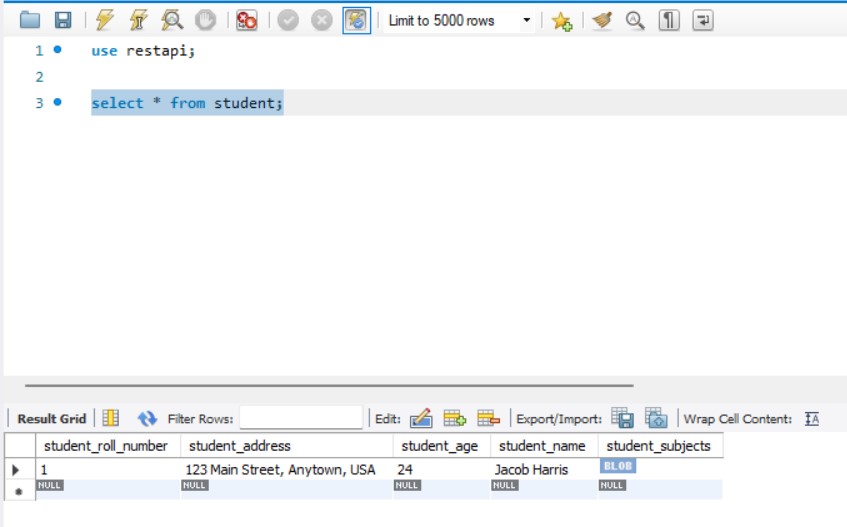
Endpoint 4: DELETE – http://localhost:8080/ students/1
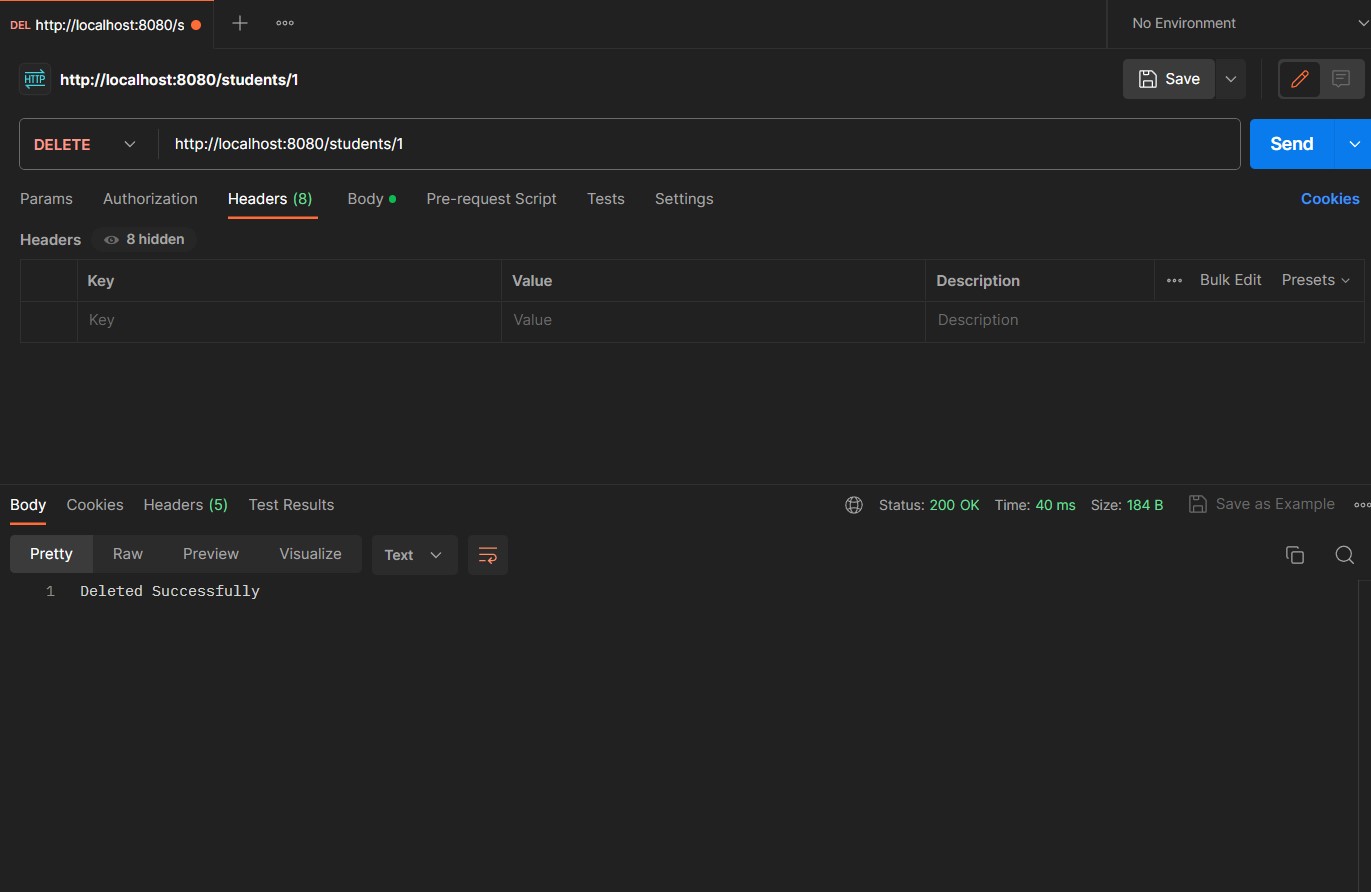
Check the database if the value is deleted as shown in the image below.
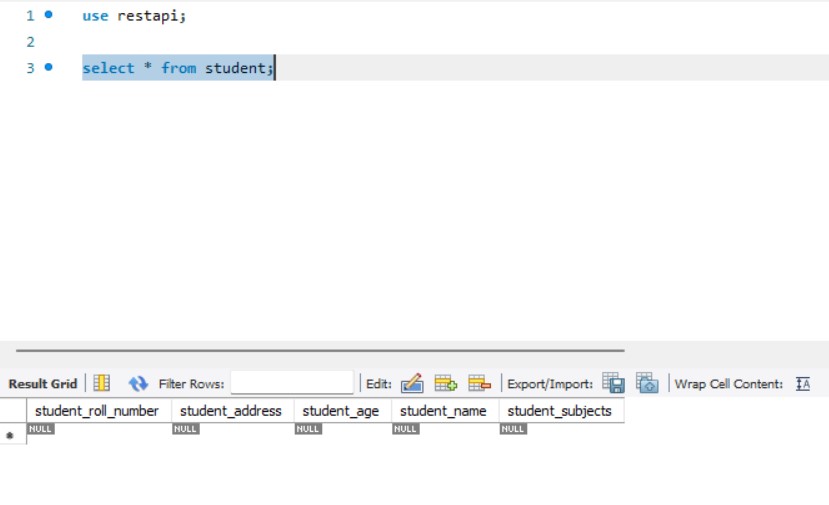
Conclusion
In this hands-on, we learned how to create a REST API with Spring Boot. This API helps us do basic things like adding, reading, updating, and removing data in a MySQL database. We used useful tools like Spring Boot’s CrudRepository and Lombok.
First, we started by setting up a new Spring Boot project using Spring Boot Initializr and opened it in IntelliJ IDE. We organized our work into different parts and made sure our code was clean and well-organized.
Then, we added the necessary libraries to our project and wrote our code. After that, we tested it to make sure it worked correctly. To do this, we used the Postman tool. We also checked the database using SELECT queries to see if the data we added was there.
We will come up with more such use cases in our upcoming blogs.
Meanwhile…
If you are an aspiring Java developer and want to explore more about the above topics, here are a few of our blogs for your reference:
- How to build Spring Boot FreeMarker Form Validation?
- How to Analyze Java Class at Runtime Using Java Reflection API?
- How to write Clean Code with Dependency Injection in Java?
Stay tuned to get all the updates about our upcoming blogs on the cloud and the latest technologies.
Keep Exploring -> Keep Learning -> Keep Mastering
At Workfall, we strive to provide the best tech and pay opportunities to kickass coders around the world. If you’re looking to work with global clients, build cutting-edge products, and make big bucks doing so, give it a shot at workfall.com/partner today!