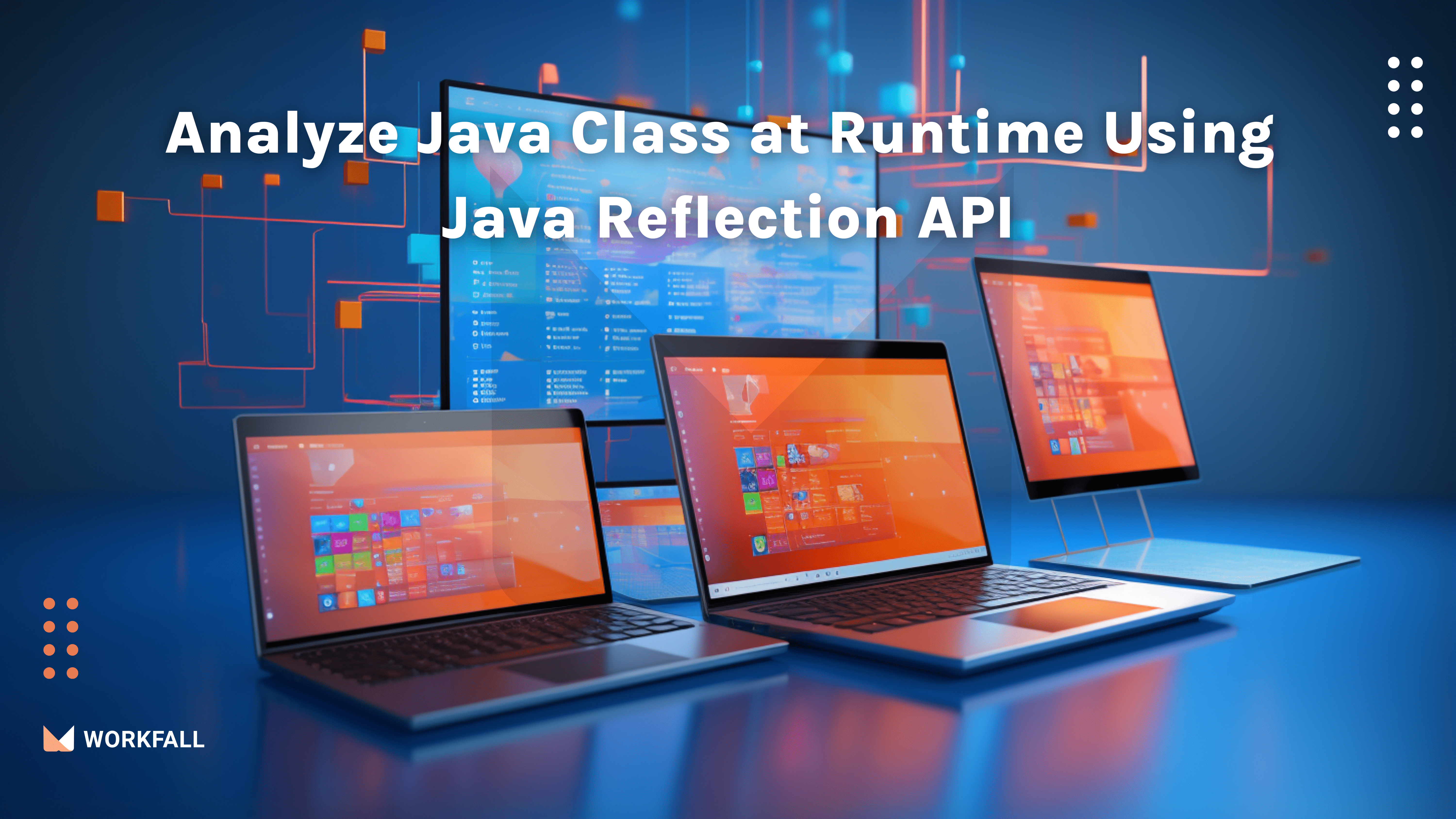
What is Reflection API?
Reflection API is one of the best features in Java. A programmer can use this API to write any logic for classes that will be generated in the future. In simple words, it refers to the ability of a running Java program to look at itself and understand its own internal details. It allows the program to examine and access information about its own components, such as the names of its variables and functions. It’s like a program looking in the mirror to see what it’s made of and how it functions.
For example: In JavaBeans, reflection is a helpful technique used by builder tools to work with software components visually. The builder tool can understand and manipulate the properties of Java components (classes) as they are loaded into the program. It’s like the builder tool has a special “magic eye” to see how each component works and can change its properties on the go, making it easier to build and customize software components visually.
Hands-On
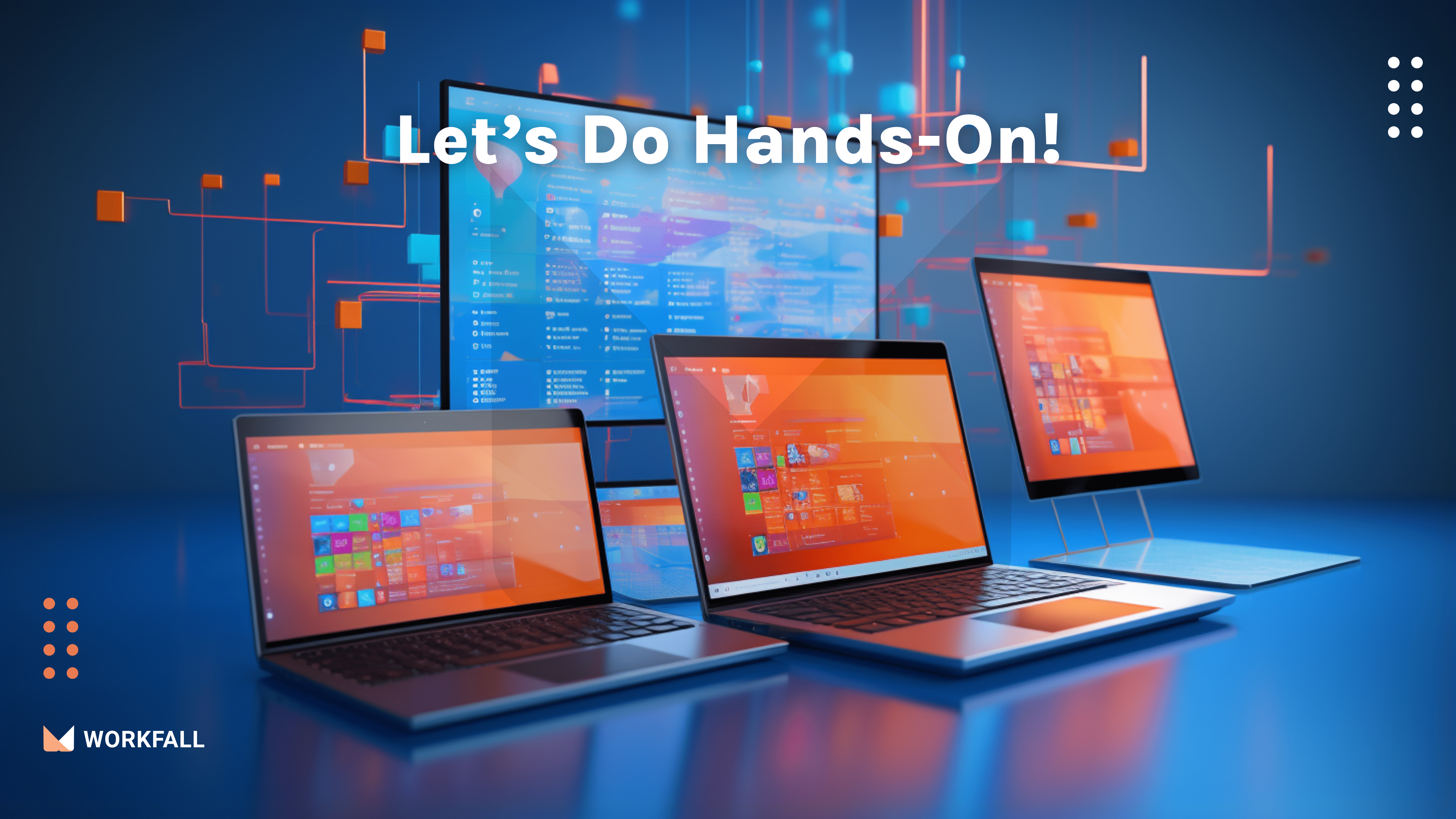
Required installations:
To perform the demo, you require the following installations:
- Visual Studio Code (IDE): It is a feature-rich source code editor designed to enhance coding speed and efficiency by offering a wide range of useful tools.
- Java: Java is a popular, object-oriented programming language known for its platform independence. It’s widely used for building diverse applications, from web and mobile apps to backend services. Java’s strong libraries, ecosystem, and portability make it a cornerstone of modern software development.
In this hands-on, we will learn how to use Reflection API to provide a way to inspect and manipulate the structure, behavior, and attributes of classes, interfaces, methods, fields, and other program entities at runtime. Reflection lets you invoke methods by name, which can be useful for generic code, scripting engines, and dynamic method dispatch.
For this we will create 2 classes one will be a class that will contain fields, properties, and methods and the second one will be the analyzer class which will analyze the first class at runtime and will determine how many functions/methods, properties first class has.
We will see different methods of loads class and getting instances of any particular class. By following these steps, you are about to embark on an enjoyable and rewarding journey of using Reflection API which is covered in this tutorial.
For this first we will need to install Java so follow the steps mentioned below: You can ignore these steps if Java is installed already.
Installation Of Java:
Step 1: Verify that Java is installed or not.
We want to determine if Java is currently installed on your local machine. Since it’s not installed, we need to download and set up JDK 17.
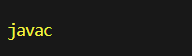
Step 2: Download JDK
Refer to the below link to download JDK 17 for your Windows 64-bit system:
https://www.oracle.com/in/java/technologies/downloads/#java17
Step 3: Install JDK
Open the file you’ve just downloaded that can be run, and then follow the instructions provided.
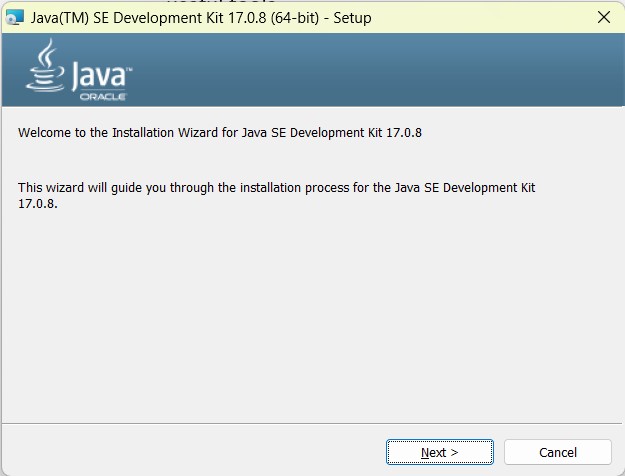
Choose the Destination folder in which you want to install JDK. Click Next to continue with the installation.
Click Next to continue.
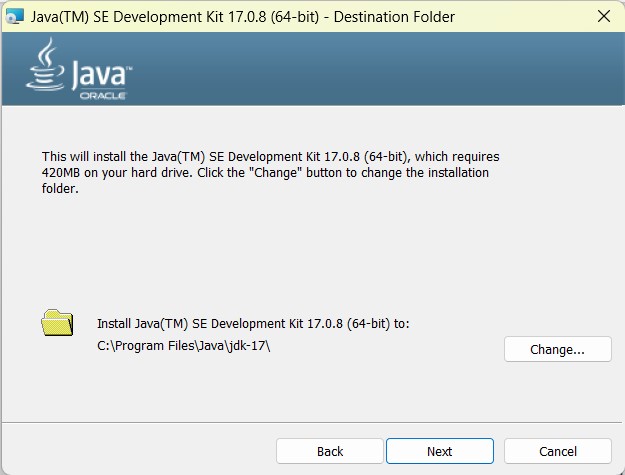
The setup is installing Java on the computer.
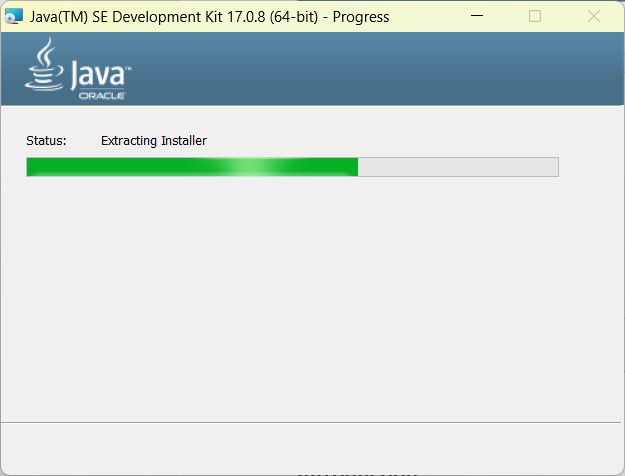
We have successfully installed Java SE development kit 17. Close the installation setup.
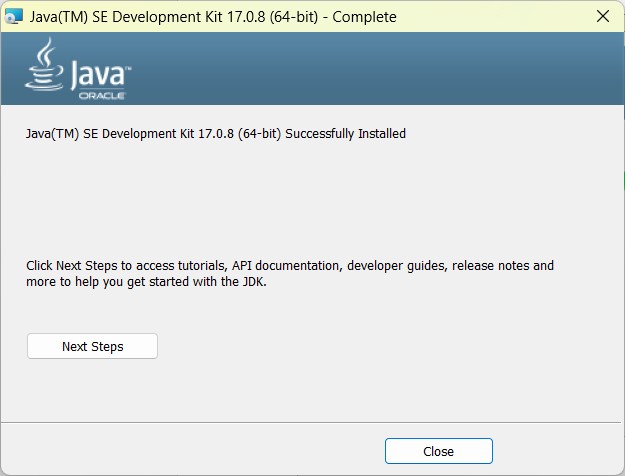
Step 4: Set the Permanent Path
To run Java programs using the command line, we have to configure the Java Path. To do this, go through the steps below.
Click with your right mouse button on “this PC,” which might be labeled as “My Computer” on certain systems. Then, select “properties” from the available choices.
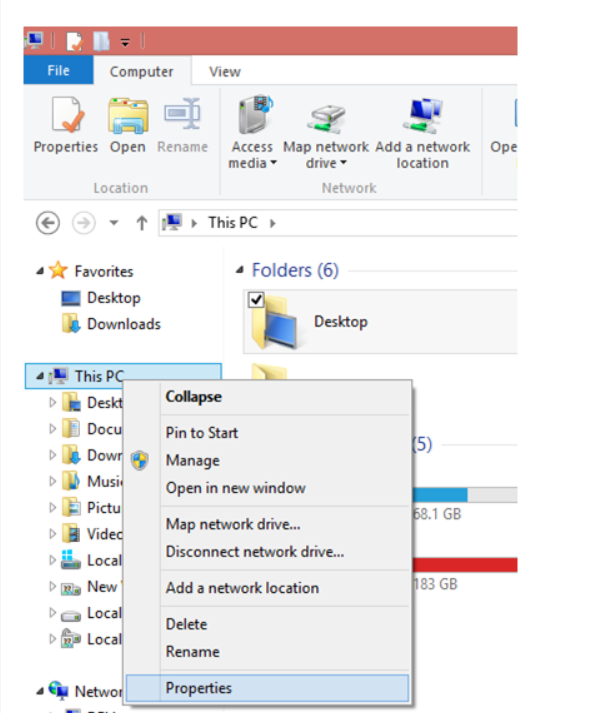
Search for advanced System Settings to continue.
Below window will open. Click on “Environment Variables” to continue.
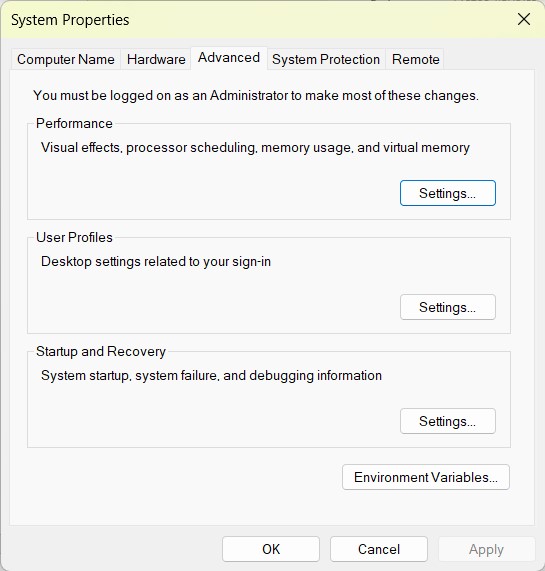
Click on the New Button in System Variables.
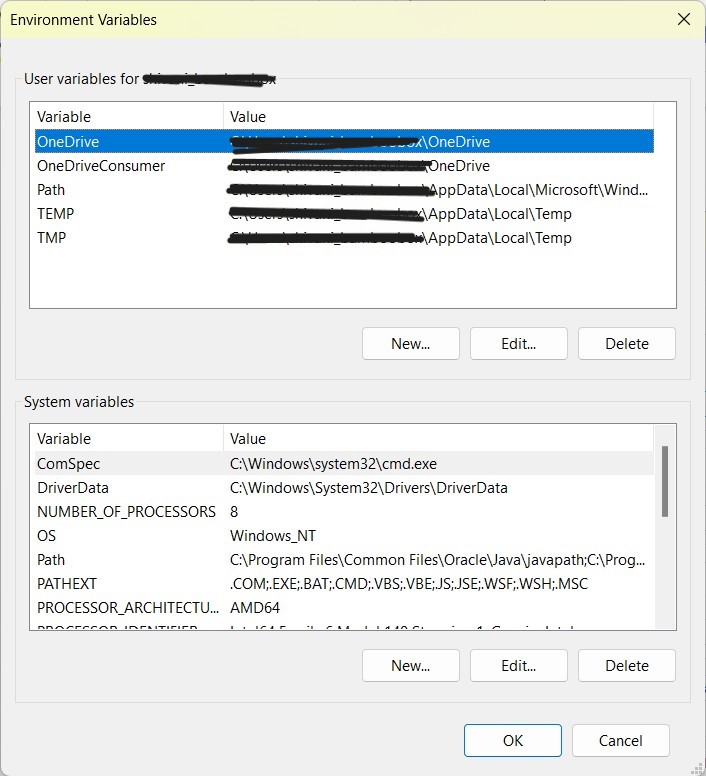
Type “path” as the variable name and input the path to the “bin” folder within your JDK in the variable value. Click OK.
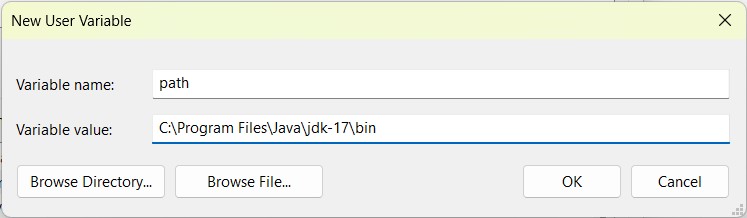
Now your Java Path is configured. Launch the Command Prompt and enter “javac.” If you already have the Command Prompt open, we recommend closing the current window and reopening it.
Type the following command to check whether Java is installed successfully or not.
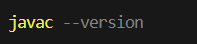
You will get the following output.

Now we will start with the practical part
Create a new directory on your local machine. Here we created it with the name Reflection API.
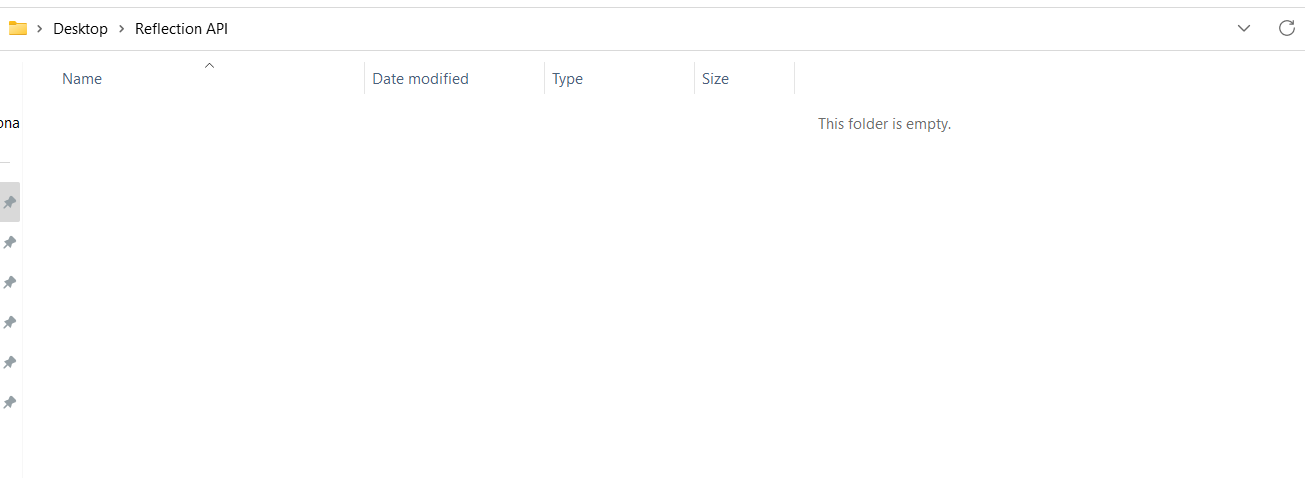
Open VS Code and click on open, to open the directory you just created.
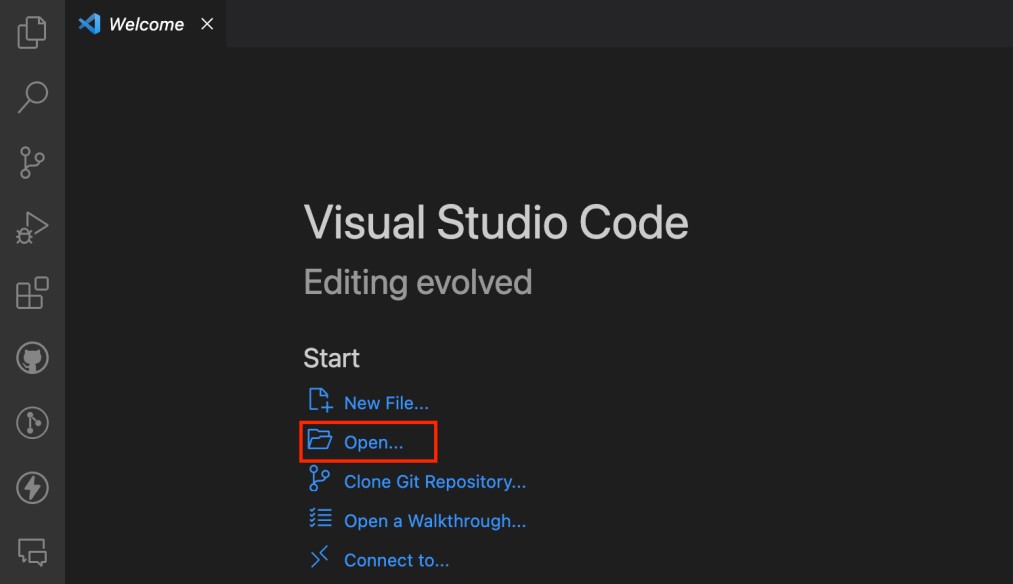
After opening the newly created directory, you will see a welcome screen like this.
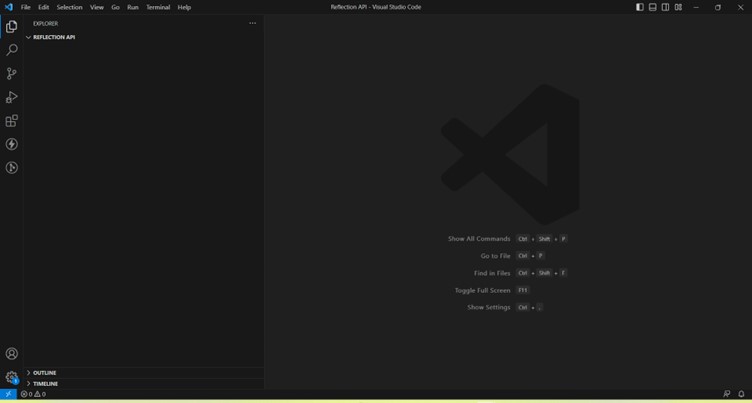
Now, in the folder structure on the left side create files to do the practical.
Setting Up to Use Reflection
The java.lang.Class class serves two main purposes:
- It offers methods to access a class’s details while the program is running. This means you can gather information about a class dynamically.
- It provides methods to inspect and modify how a class behaves while the program is running. This allows you to understand and adjust the way a class works during execution
In reflection API we load a class and then we can determine how many functions does class has, what is the return type of the function, what are the parameters of the function, what are fields the class contains, etc.
The reflection classes, like Method, are located in java.lang.reflect. To utilize these classes, you should follow three steps.
- The initial step involves acquiring a java.lang.Class object for the specific class you intend to handle. The java.lang.Class is employed to symbolize classes and interfaces within an active Java program.
How to get the object of the Class class?
There are 3 ways to get the instance of Class class. They are as follows:
- forName() method of Class class
- getClass() method of Object class
- the .class syntax
1)forName() method of Class class
Is used for dynamic class loading. Yields an instance of the Class class. It’s suitable when you’re aware of the complete class name. However, this approach isn’t applicable to primitive types.
FileName: Example1.java
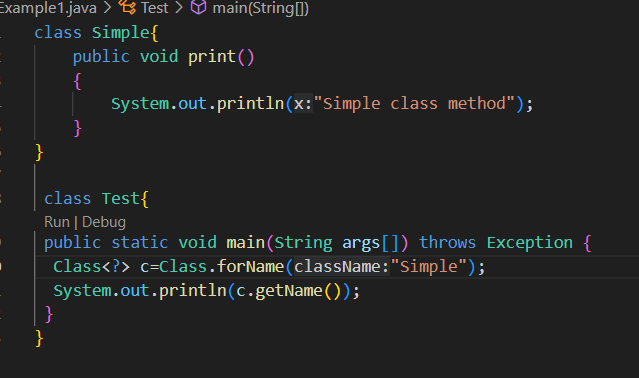
The Test class dynamically loads the class named “Simple” using Class.forName(“Simple”). This enables the code to work with classes that aren’t known at compile time. It then retrieves the loaded class’s name using c.getName() and prints it. Here forName method will return the instance of Class which is Simple.
Run the below-provided command to compile the above code.

Run the below-provided command to run the above code.
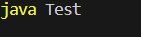
You will get the below output.

2) getClass() method of Object class
It returns the instance of the Class class. It should be used if you know the type. Moreover, it can be used with primitives.
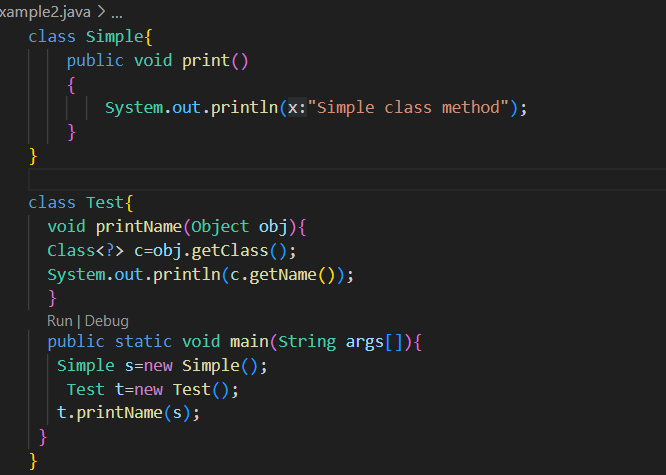
The Test class contains a method called printName(Object obj) that takes an argument of type Object. Inside this method:
• It retrieves the class of the provided object using the getClass() method.
• It prints the name of the class using the getName() method of the Class object.
Run the below-provided command to compile the above code.

Run the below-provided command to run the above code.
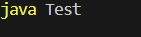
You will get the below output.

3) The .class syntax
If a type is available, but there is no instance, then it is possible to obtain a Class by appending “.class” to the name of the type. It can be used for primitive data types also.
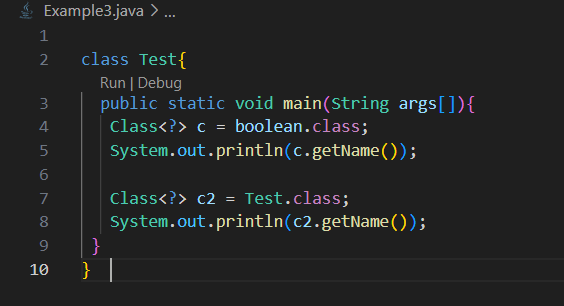
The code demonstrates how to obtain and print class names using getName(). This showcases the capability to reference and display class names dynamically.
Run the below-provided command to compile the above code.

Run the below-provided command to run the above code.
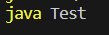
You will get the below output.
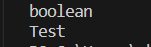
Concept-: First load a class and then analyze it.
The second step is to call a method such as getDeclaredMethods, to get a list of all the methods declared by the class.
Once this information is in hand, then the third step is to use the reflection API to manipulate the information. For example, the sequence:
Class c = Class.forName("java.lang.String");
Method m[] = c.getDeclaredMethods();
System.out.println(m[0].toString());
We will display a textual representation of the first method declared in String.
Refer to the below example to see how Reflection API works:
Here there is a class named Student.java which has setter and getter methods and properties which we will analyze at runtime using reflection API to explore.
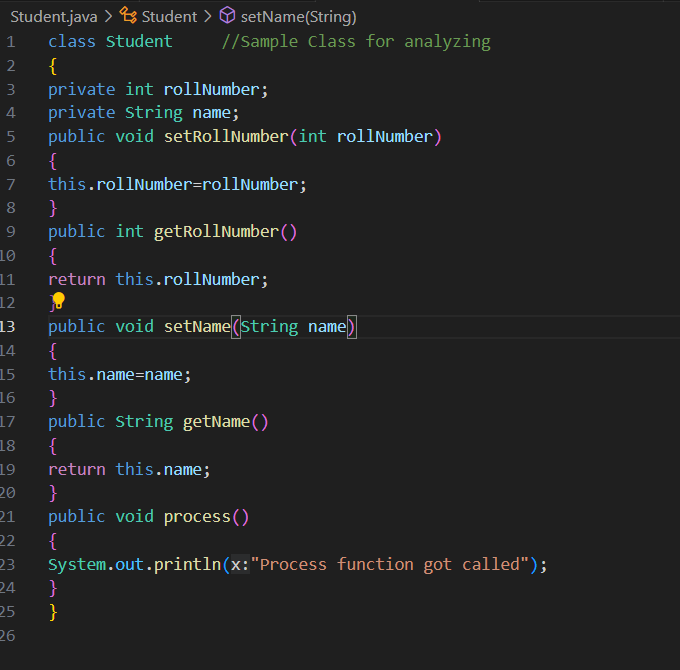
Run the below-provided command to compile the above code.

Now we will create an Analyzer class that will accept a class name using command line arguments which will analyze the class at runtime. The reflection API package is java.lang.reflect.
So first we will import this package and check the length of the class which will be provided in command line arguments. If the length is not equal to 1 we will return from here.
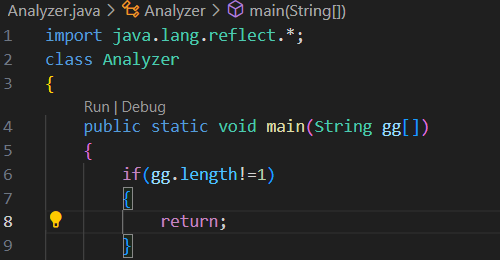
Now use forName method which will return the instance of Class and then will call getName() which will return the className with the package included in it.
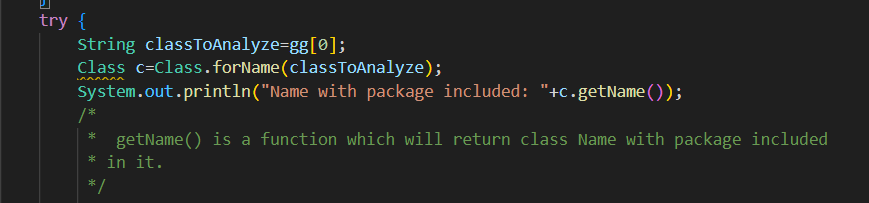
We can use the getSimpleName() method which will return the simple name of the class in the form of a string. This method does not accept any parameter.

The getDeclaredMethods() method is provided by the Class class in Java’s reflection API, and it returns an array of Method objects representing all methods declared in the class.
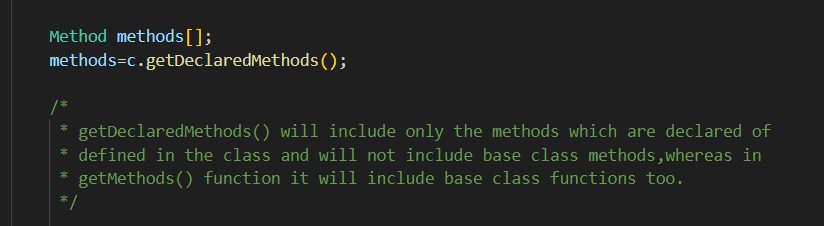
Now we will retrieve an array of methods from a class. It iterates through each method, extracting its name and printing it to the console. The loop iterates through the array of Method objects, assigning each method to the variable m. The getName() method fetches the method’s name, which is then displayed on the console.
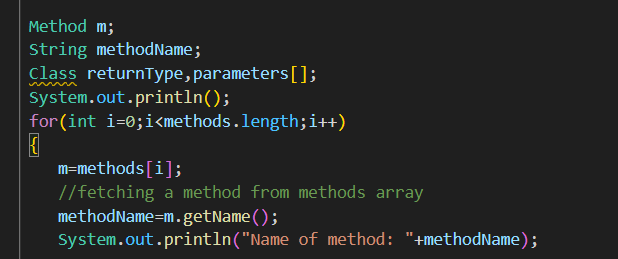
getReturnType() method retrieves and stores the method’s return type. The code then displays the return type information for each method in the class.

getParameterTypes() method is utilized to retrieve an array representing the parameter types of each method in a class.

Now the loop iterates through the parameters array, representing the parameter types for the current method.
The code prints the parameter’s index (position) and its name using the getName() method of the Class object which allows the dynamic display of parameter information for each method, offering insight into method signatures and parameter types during runtime through reflection.

Now there is another method which is getDeclaredFields() which will return the information related to the fields that are declared in the class.
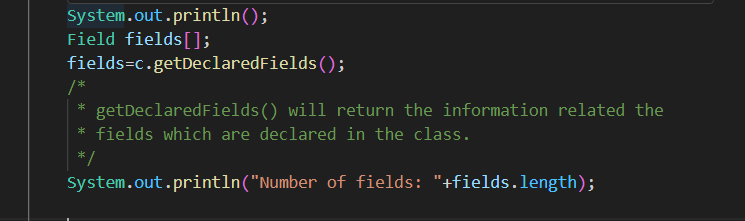
The below lines of code iterate through an array of fields within a class. For each field, it retrieves the field’s name and type using reflection.
The code then prints the field’s position, name, and type name to the console, providing insight into the class’s field structure at runtime.
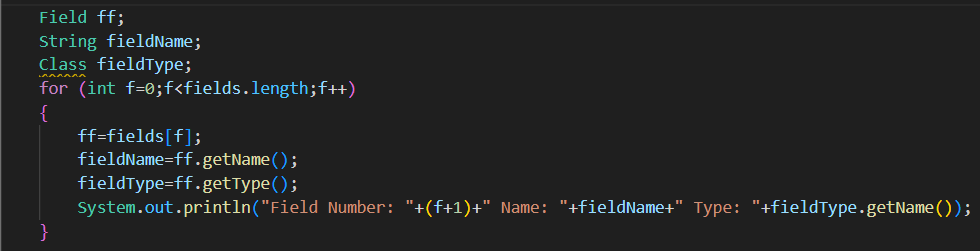
If a class is not found during runtime, the code catches a ClassNotFoundException and prints a message indicating the missing class.
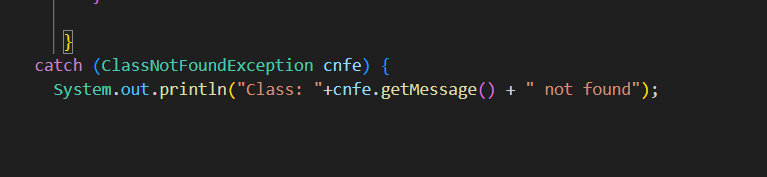
Run the below-provided command to compile the above code.

Run the below-provided command to run the above code.

You will get the below output.
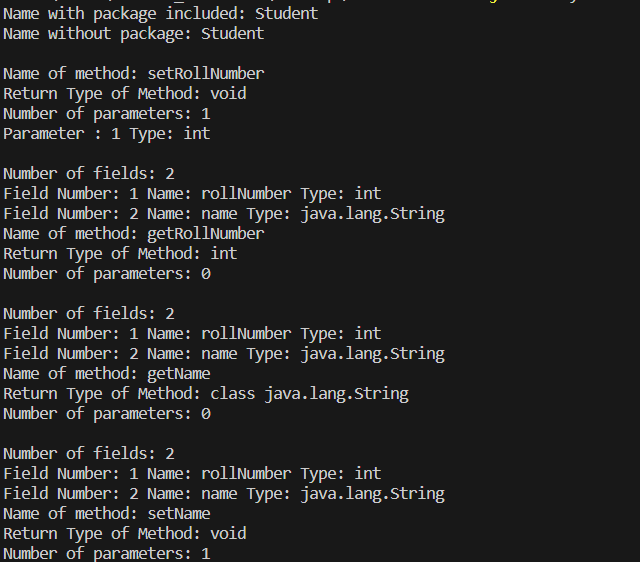
Conclusion
In this hands-on, we have learned using Reflection API we can create magical things as we can analyze any class that will be created by any programmer in the future.
Java reflection is valuable because it enables the dynamic retrieval of details about classes and data structures based on their names. Moreover, it facilitates their manipulation within a running Java program. This capability is remarkably potent and doesn’t have a counterpart in other traditional languages like C, C++, Fortran, or Pascal.
We will come up with more such use cases in our upcoming blogs.
Meanwhile…
If you are an aspiring Java developer and want to explore more about the above topics, here are a few of our blogs for your reference:
- How to create test cases and start testing the JavaScript code using Mocha?
- How to write Clean Code with Dependency Injection in Java?
Stay tuned to get all the updates about our upcoming blogs on the cloud and the latest technologies.
Keep Exploring -> Keep Learning -> Keep Mastering
At Workfall, we strive to provide the best tech and pay opportunities to kickass coders around the world. If you’re looking to work with global clients, build cutting-edge products, and make big bucks doing so, give it a shot at workfall.com/partner today!