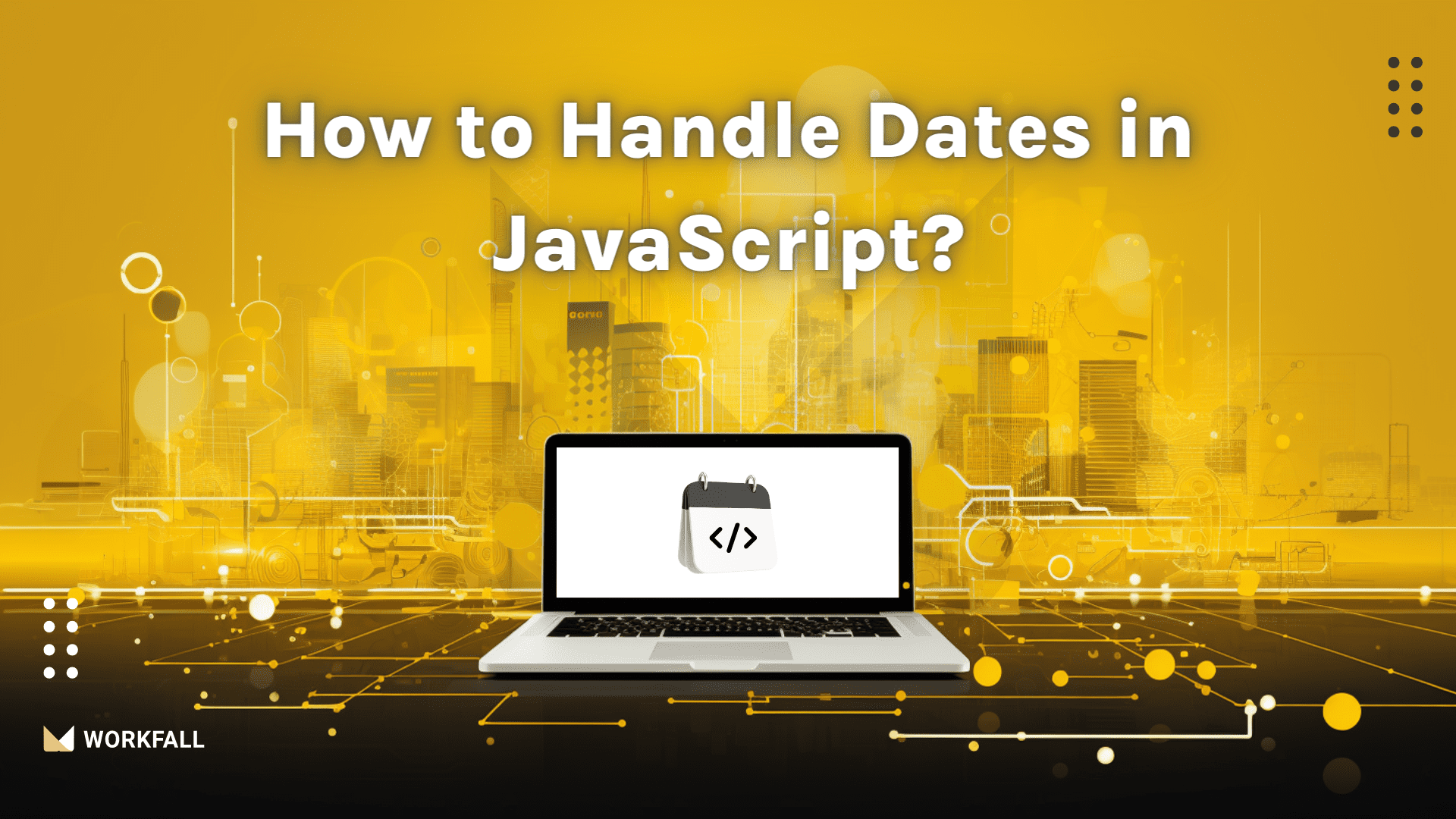
In this blog, we’ll explore the fascinating realm of JavaScript’s Date object by showing a step-by-step implementation of how to handle dates in JavaScript. We’ll dig deep into its importance in web development, specifically how it helps manage time on the user’s device.
Instead of relying on servers, JavaScript’s Date object allows us to handle dates and times directly within web browsers. Basically, this is a key element in creating interactive and responsive web interfaces. We’ll uncover how it influences various aspects of web development.
Why Do We Need Javascript Date?
JavaScript’s `Date` object is essential for several reasons in web development and other programming contexts:
- Client-Side Time Handling: JavaScript runs in web browsers, which are primarily used for client-side scripting. It allows you to work with dates and times on the client-side, making it possible to display and manipulate dates without requiring server-side interactions. This is crucial for building dynamic and responsive user interfaces.
- User Interaction: JavaScript is commonly used for building interactive web applications. The `Date` object enables developers to manage events and actions related to dates and times, such as date pickers, countdown timers, and scheduling features.
- Displaying Dynamic Content: Many websites and web applications display content that changes over time. JavaScript’s `Date` object allows developers to dynamically update and render time-sensitive data on web pages, such as news articles with publication dates, social media posts with timestamps, and live clocks.
- Data Manipulation and Validation: JavaScript’s `Date` object provides methods for parsing and formatting date strings, making it useful for validating and processing user-inputted dates. It allows you to ensure that dates meet specific criteria and convert them to a consistent format.
- Event Scheduling: JavaScript can be used to schedule events and actions to occur at specific times or intervals. This is important for building features like reminders, notifications, and automated tasks.
- Time Zone Handling: The `Date` object provides mechanisms for handling time zones, which is crucial when dealing with users from different regions and ensuring that dates and times are displayed accurately and consistently.
- Calculations and Comparisons: JavaScript’s `Date` object allows for date arithmetic, making it possible to calculate durations, find the difference between two dates, and perform comparisons between dates. This is useful for tasks like calculating age, time elapsed, or determining if a date is in the past or future.
Hence, the `Date` object in JavaScript is a fundamental tool for working with dates and times, enabling developers to create dynamic and time-sensitive web applications, handle user interactions, and ensure accurate date-related functionality across various use cases.
Hands-On
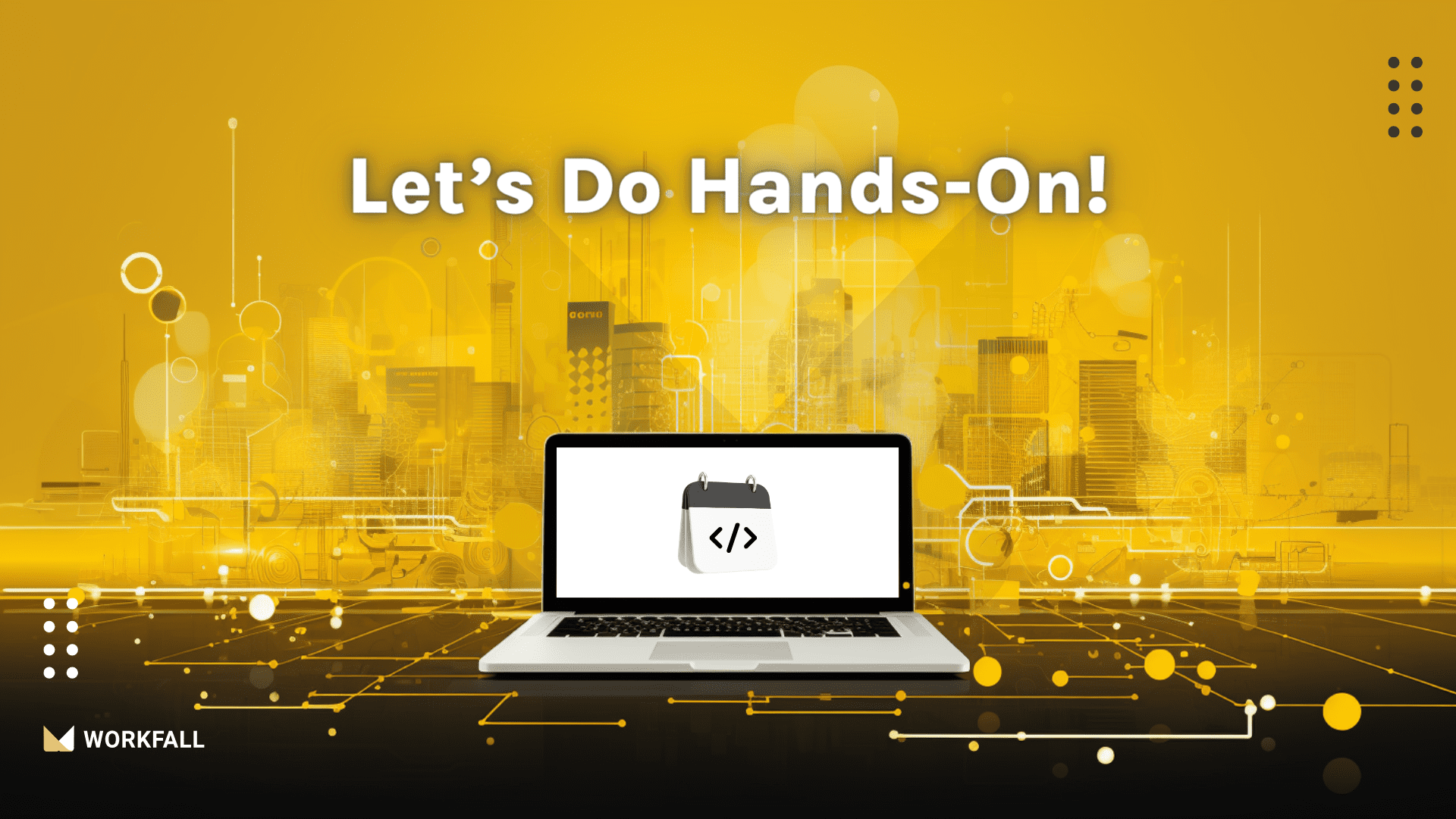
In this hands-on, we are going to learn a wide range of functions, each building on what we’ve learned before to give you a solid grasp of working with dates and times. We’ll start by understanding time zones.
Then, we’ll go to the basics, like creating date objects in JavaScript. We’ll learn how to make dates using different pieces of information, like specific arguments, timestamps, or even without any details, giving you the flexibility to handle time-related data.
As we move forward, we’ll dive into formatting dates, so your date representations look just the way you want them. You’ll also find out how to create custom date formats for specific needs.
Comparing dates will be a breeze as we explore ways to figure out how different dates relate to each other. You’ll get the hang of extracting dates from one another and doing date math, like adding or subtracting time.
Lastly, we’ll talk about automatic date correction, making sure your date and time data always stays accurate. With each step, you’ll build the skills to confidently work with dates and times in JavaScript, making your web applications more functional and user-friendly.
Time Zones
In JavaScript, we mainly deal with two time zones: Local Time and Coordinated Universal Time (UTC).
- Local Time is the timezone of your computer, where you are currently located.
- UTC is similar to Greenwich Mean Time (GMT).
By default, most JavaScript date methods provide dates and times in your local time zone. You only get UTC time if you specifically ask for it.
Now, let’s talk about how to create dates.
Creating a date
You can make a date using `new Date()`. There are four ways to do it:
- With a date-string
- With date arguments
- With a timestamp
- With no arguments
The date-string method
In the “date-string” method, you make a date by giving a date as a string to the new Date function.

We often prefer using date strings to write dates, which feels natural because we’ve used them for a long time.
But if I write “14-03-2012” you can easily understand that it means the 14th of March, 2012. However, in JavaScript, if you enter “14-03-2012” as a date, it will show as an “Invalid Date”.
There’s a clear reason for this.
Date strings can be understood differently in different places. For instance, “10-02-2021” could mean either February 10, 2021 or October 2, 2021. It’s hard to tell which one it is unless you know the specific date format being used.
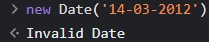
When using a date string in JavaScript, it’s important to choose a format that works everywhere. One such widely accepted format is the ISO 8601 Extended format.

Here’s what those values mean:
- YYYY: The year in four digits.
- MM: The month is in two digits, where January is 01 and December is 12.
- DD: The date is in two digits, from 0 to 31.
- -: These are the date separators.
- T: It shows the beginning of the time part.
- HH: The hour in 24-hour format, from 0 to 23.
- mm: Minutes from 0 to 59.
- ss: Seconds from 0 to 59.
- sss: Milliseconds from 0 to 999.
- :: These are the time separators.
- Z: If you see Z, it means the date is in UTC time. If there’s no Z, it’s in local time (if time is provided).
You don’t have to specify hours, minutes, seconds, or milliseconds when making a date. For example, to create a date for February 10, 2021, you can simply write:

Creating dates using arguments
You can create a date/time in JavaScript by providing up to seven pieces of information:
- Year: A four-digit number representing the year.
- Month: A number indicating the month of the year (0-11). The first month is represented as 0. If you don’t specify it, it’s assumed to be 0.
- Day: A number representing the day of the month (1-31). If you don’t provide this, it’s set to 1.
- Hour: A number indicating the hour of the day (0-23). If you don’t mention it, it’s set to 0.
- Minutes: A number representing the minutes (0-59). If left out, it’s considered as 0.
- Seconds: A number for the seconds (0-59). If not given, it’s set to 0.
- Milliseconds: A number for the milliseconds (0-999). If not specified, it’s set to 0.

Many developers sometimes steer clear of the “arguments approach” because it might seem complex at first glance. However, it’s simpler than it appears.
Think of it like reading numbers from left to right. As you move from left to right, you insert values in decreasing order of importance: year, month, day, hours, minutes, seconds, and milliseconds.

Remember that the month is zero-indexed in JavaScript dates.
Here are some additional examples for you to get to know better:
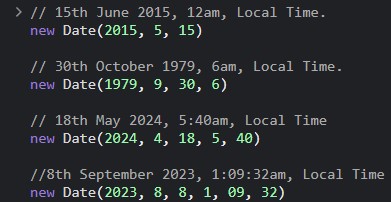
When you create dates using arguments, they are automatically set to your local time, which means the time zone of your computer or device. This can be handy because it avoids confusion between local time and UTC (Coordinated Universal Time). However, if you ever need a date in UTC, you can create one like this:

Creating dates with timestamps
In JavaScript, a timestamp measures the time in milliseconds that has passed since a specific date known as the “Unix epoch time,” which is January 1, 1970. Typically, you don’t use timestamps to create dates; instead, you use them mainly for comparing different dates, which we’ll discuss more in detail later.

With no arguments
If you make a date with no arguments, you’ll get a date that shows the current time in your local time zone.

Summary of Creating Dates
Creating dates in JavaScript is straightforward using the new Date() method.
There are four ways to do it:
- Using a Date String: You can create a date by providing a date string, but it’s not the recommended method.
- Using Arguments: It’s best to create dates by passing specific arguments like year, month, day, etc. However, remember that in JavaScript, months are zero-indexed.
- Using Timestamps: You can also create dates by providing a timestamp.
- With No Arguments: If you want the current date and time, simply create a date with no arguments.
Avoid using the date string method and prefer creating dates with arguments. Just keep in mind that months start from 0 in JavaScript.
Next, we’ll learn how to convert a date into a readable string.
Formatting a date
Many programming languages offer a handy way to create custom date formats. In PHP, you can use date(“d M Y”) to format a date as something like “27 Feb 2023.”
The native Date object provides seven formatting methods, and each of these methods gives you a particular value.
Let’s suppose we have a date object as shown in the image below:

Let’s see each of the methods in action.
- toString()

- toDateString():
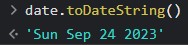
- toLocaleString():

- toLocaleDateString():

- toGMTString():

- toUTCString():

- toISOString():

To have a format that’s just right for you, you’ll have to make it yourself.
Creating your own date format
Suppose you want a date like “Sun, 24 September, 2023”. To make this, you’ll need to understand and use the built-in date methods that come with the Date object.
To obtain dates, you can make use of these four methods:
1. getFullYear: It fetches the four-digit year based on your local time.
2. getMonth: This method retrieves the month of the year, using a numbering system from 0 to 11, where January is 0 and December is 11.
3. getDate: It retrieves the day of the month, ranging from 1 to 31, based on your local time.
4. getDay: This method fetches the day of the week, represented from 0 to 6. The week starts with Sunday as 0 and ends with Saturday as 6.
You can easily obtain “24” and “2023” for “Sun, 24 September 2023” by using the functions getFullYear() and getDate() as shown in the image below.
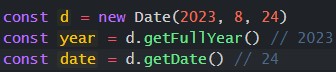
Getting Sun and September is more challenging.
To get September, you have to make something that connects all twelve months with their names.
Since months are counted starting from zero, we can use an array to represent them.
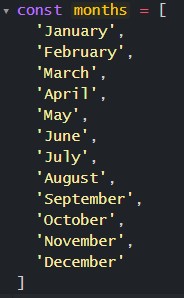
To find out which month is September, follow these steps:
- Use “getMonth()” to get the month number starting from zero in the date.
- Then, match that month number with the month name in the list of months.
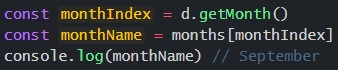
To find the Sun, follow the same steps. This time, you’ll need a list with all seven days of the week.
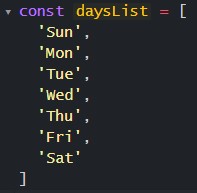
We need to get the dayIndex with the getDay() method and use the dayIndex to get the day name as shown in the image below.
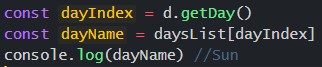
Next, we need to bring together all the variables we made to create the formatted string. See the image below.

The final code will look something like shown in the image below.
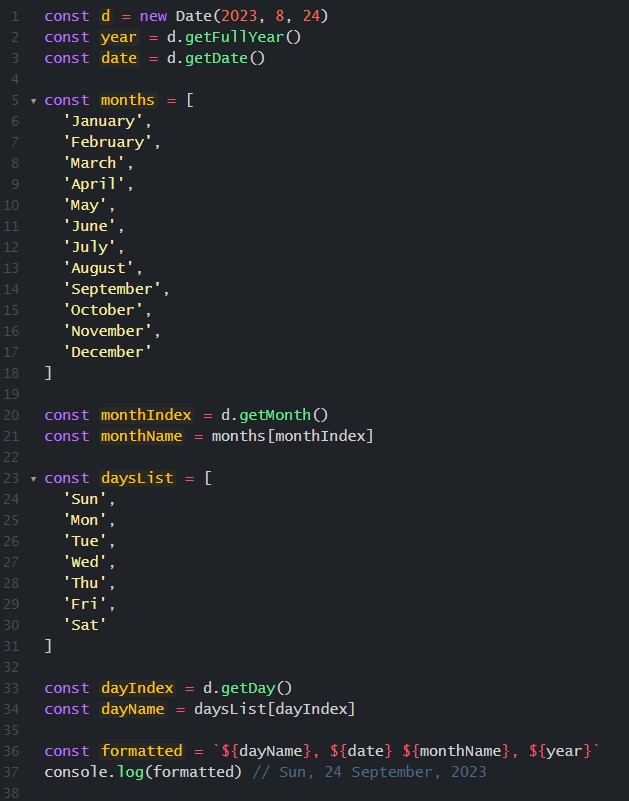
When you want to make a time look exactly how you want it, you can use these methods:
- getHours: It gives you the hours (from 0 to 23) in your local time.
- getMinutes: This one gets you the minutes (from 0 to 59) in your local time.
- getSeconds: It fetches the seconds (from 0 to 59) in your local time.
- getMilliseconds: This one’s for the milliseconds (from 0 to 999) in your local time.
Now, let’s move on to comparing dates.
Comparing dates
To find out if one date is earlier or later than another, you can simply use symbols like > (greater than), < (less than), >= (greater than or equal to), and <= (less than or equal to) to compare them.
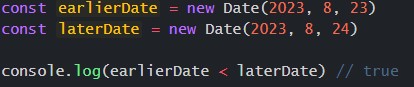
Checking if two dates occur at precisely the same time can be tricky. Using “==” or “===” for comparison won’t work in this case.
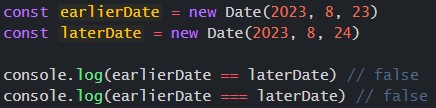
To see if the two dates are exactly the same, we can compare their timestamps using the “getTime()” method as shown in the image below.
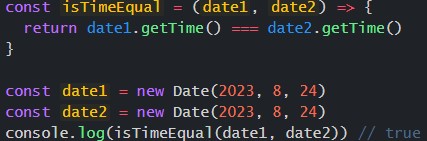
To see if two dates are on the same day, we can compare their year, month, and day values using “getFullYear”, “getMonth”, and “getDate” as shown in the image below.
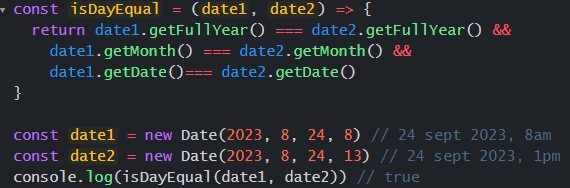
Getting a date from another date
There are two situations where you might need to work with dates based on other dates:
- Copying a Date/Time: You want to make one date have the same date and time as another date.
- Adjusting by an Amount: You need to change a date by a certain amount, either adding to it or subtracting from it.
Setting a specific date/time
You can change a date or time using these methods:
setFullYear: Change the year (using 4 digits) in your local time.
setMonth: Modify the month of the year in your local time.
setDate: Adjust the day of the month in your local time.
setHours: Update the hours in your local time.
setMinutes: Alter the minutes in your local time.
setSeconds: Change the seconds in your local time.
setMilliseconds: Adjust the milliseconds in your local time.
For example, if you want to make a date fall on the 8th day of the month, you can use setDate(8).
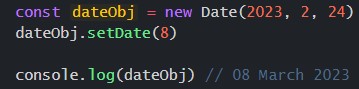
To make the month September, you can use the “setMonth” method. Just remember that months start from zero, not one. So, September would be represented as 8, not 9.
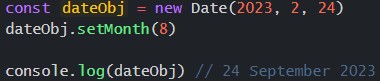
Adding or subtracting a delta from another date
A “delta” simply means a change. When we talk about adding or subtracting a delta from another date, we mean this: you want to figure out a new date that’s a certain amount different from the original date. This difference could be in years, months, days, or any other unit of time.
To calculate this difference, you need to know the current date’s values, which you can find using these methods:
getFullYear: This gives you the year as a four-digit number, according to your local time.
getMonth: It tells you the month of the year, ranging from 0 to 11, based on your local time.
getDate: This provides the day of the month, from 1 to 31, according to your local time.
getHours: It gives you the hour of the day, from 0 to 23, based on your local time.
getMinutes: This tells you the minutes, from 0 to 59, according to your local time.
getSeconds: It gives you the seconds, from 0 to 59, based on your local time.
getMilliseconds: This provides the milliseconds, from 0 to 999, according to your local time.
Let’s assume today is 24th September 2023

To start, we make a fresh Date object.

Next, we need to figure out what we want to modify. In this case, we’re changing days, so we can find out the current day using “getDate()”.

We need to find a date that’s ten days in the future. To do this, we’ll simply add ten days to the current date.

The final code should look as shown in the image below.
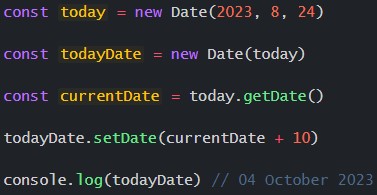
Automatic date correction
If you give JavaScript a date that doesn’t make sense, like September 35, 2023 (there’s no September 35 on the calendar), JavaScript will fix it for you. In this case, it changes September 35 to October 5 automatically.

You don’t have to do the math for minutes, hours, days, or months when creating a time difference. JavaScript takes care of it for you without any extra effort.

Conclusion
In this hands-on, we’ve explored a wide range of functions related to date objects in JavaScript to give you a strong understanding of working with dates and times.
We started by understanding time zones, which is crucial when dealing with global applications.
Afterwards, we delved into the basics, like creating date objects in JavaScript. We learned how to make dates using different pieces of information, providing you with flexibility in handling time-related data.
Furthermore, we explored formatting dates, ensuring your date representations match your needs. You also discovered how to create custom date formats for specific requirements.
Comparing dates became second nature as we explored ways to figure out how different dates relate to each other. You gained the ability to extract dates from one another and perform date math, like adding or subtracting time.
Lastly, we discussed automatic date correction, ensuring your date and time data always stays accurate. With each step, you’ve built the skills to confidently work with dates and times in JavaScript, making your web applications more functional and user-friendly. We will come up with more such use cases in our upcoming blogs.
Meanwhile…
If you are an aspiring Frontend developer and want to explore more about the above topics, here are a few of our blogs for your reference:
- How to create test cases and start testing the JavaScript code using Mocha?
- How to Get the User’s Location Using Mapbox?
Stay tuned to get all the updates about our upcoming blogs on the cloud and the latest technologies.
Keep Exploring -> Keep Learning -> Keep Mastering
At Workfall, we strive to provide the best tech and pay opportunities to kickass coders around the world. If you’re looking to work with global clients, build cutting-edge products, and make big bucks doing so, give it a shot at workfall.com/partner today!